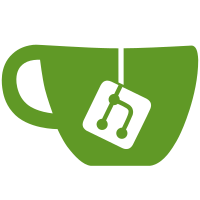
no issue - our API always returns an array whether we're performing a browse or find request but Ember Data expects explicit find requests to return a single object and throws deprecations when it sees an array - https://deprecations.emberjs.com/ember-data/v2.x/#toc_store-queryrecord-array-response-with-restserializer - we previously had `normalizeSingleResponse` overrides in specific models that we use with `queryRecord` but we've since introduced `queryRecord` usage on more models but the associated "fix" was not duplicated in the serializers for those models leading to many deprecation warnings logged to the console in development and when testing - moved the fix to the application serializer so it applies to all models - explicitly excluded `setting` model because that's a special-case and has it's own array-into-object serialization to represent multiple settings records as a single model instance
65 lines
2.1 KiB
JavaScript
65 lines
2.1 KiB
JavaScript
import RESTSerializer from '@ember-data/serializer/rest';
|
|
import {camelize, decamelize, underscore} from '@ember/string';
|
|
import {pluralize} from 'ember-inflector';
|
|
|
|
export default class Application extends RESTSerializer {
|
|
// hacky method for getting access to meta data for single-resource responses
|
|
// https://github.com/emberjs/data/pull/4077#issuecomment-200780097
|
|
// TODO: review once the record links and meta RFC lands
|
|
// https://github.com/emberjs/rfcs/blob/master/text/0332-ember-data-record-links-and-meta.md
|
|
extractMeta(store, typeClass) {
|
|
let meta = super.extractMeta(...arguments);
|
|
typeClass.___meta = meta;
|
|
return meta;
|
|
}
|
|
|
|
serialize() {
|
|
let json = super.serialize(...arguments);
|
|
|
|
// don't send attributes that are updated automatically on the server
|
|
delete json.created_by;
|
|
delete json.updated_by;
|
|
|
|
return json;
|
|
}
|
|
|
|
serializeIntoHash(hash, type, record, options) {
|
|
// Our API expects an id on the posted object
|
|
options = options || {};
|
|
options.includeId = true;
|
|
|
|
// We have a plural root in the API
|
|
let root = pluralize(type.modelName);
|
|
let data = this.serialize(record, options);
|
|
|
|
hash[root] = [data];
|
|
}
|
|
|
|
keyForAttribute(attr) {
|
|
return decamelize(attr);
|
|
}
|
|
|
|
keyForRelationship(key, typeClass, method) {
|
|
let transform = method === 'serialize' ? underscore : camelize;
|
|
|
|
if (typeClass === 'belongsTo' && !key.match(/(Id|By)$/)) {
|
|
let transformed = `${transform(key)}_id`;
|
|
return transformed;
|
|
}
|
|
|
|
return transform(key);
|
|
}
|
|
|
|
normalizeQueryRecordResponse(store, primaryModelClass, payload) {
|
|
const root = this.keyForAttribute(primaryModelClass.modelName);
|
|
const pluralizedRoot = pluralize(root);
|
|
|
|
if (payload[pluralizedRoot] && root !== 'setting') {
|
|
payload[root] = payload[pluralizedRoot][0];
|
|
delete payload[pluralizedRoot];
|
|
}
|
|
|
|
return super.normalizeQueryRecordResponse(...arguments);
|
|
}
|
|
}
|