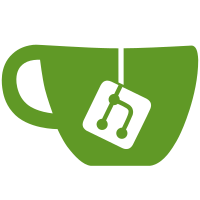
refs https://github.com/TryGhost/Product/issues/4159 --- <!-- Leave the line below if you'd like GitHub Copilot to generate a summary from your commit --> <!-- copilot:summary --> ### <samp>🤖[[deprecated]](https://githubnext.com/copilot-for-prs-sunset) Generated by Copilot at 9e68f4d</samp> This pull request refactors several components in the `admin-x-settings` app to use common hooks from the `@tryghost/admin-x-framework` package, which reduces code duplication and improves consistency. It also updates the `package.json` file and adds unit tests for the `admin-x-framework` package, which improves the formatting, testing, and dependency management. Additionally, it makes some minor changes to the `hooks.ts`, `FrameworkProvider.tsx`, and `.eslintrc.cjs` files in the `admin-x-framework` package, which enhance the public API and the linting configuration.
76 lines
2.4 KiB
TypeScript
76 lines
2.4 KiB
TypeScript
import {act, renderHook} from '@testing-library/react';
|
|
import * as assert from 'assert/strict';
|
|
import useForm from '../../../src/hooks/useForm';
|
|
|
|
describe('useForm', function () {
|
|
describe('formState', function () {
|
|
it('returns the initial form state', function () {
|
|
const {result} = renderHook(() => useForm({
|
|
initialState: {a: 1},
|
|
onSave: () => {}
|
|
}));
|
|
|
|
assert.deepEqual(result.current.formState, {a: 1});
|
|
});
|
|
});
|
|
|
|
describe('updateForm', function () {
|
|
it('updates the form state', function () {
|
|
const {result} = renderHook(() => useForm({
|
|
initialState: {a: 1},
|
|
onSave: () => {}
|
|
}));
|
|
|
|
act(() => result.current.updateForm(state => ({...state, b: 2})));
|
|
|
|
assert.deepEqual(result.current.formState, {a: 1, b: 2});
|
|
});
|
|
|
|
it('sets the saveState to unsaved', function () {
|
|
const {result} = renderHook(() => useForm({
|
|
initialState: {a: 1},
|
|
onSave: () => {}
|
|
}));
|
|
|
|
act(() => result.current.updateForm(state => ({...state, a: 2})));
|
|
|
|
assert.deepEqual(result.current.saveState, 'unsaved');
|
|
});
|
|
});
|
|
|
|
describe('handleSave', function () {
|
|
it('does nothing when the state has not changed', async function () {
|
|
let onSaveCalled = false;
|
|
|
|
const {result} = renderHook(() => useForm({
|
|
initialState: {a: 1},
|
|
onSave: () => {
|
|
onSaveCalled = true;
|
|
}
|
|
}));
|
|
|
|
assert.equal(await act(() => result.current.handleSave()), true);
|
|
|
|
assert.equal(result.current.saveState, '');
|
|
assert.equal(onSaveCalled, false);
|
|
});
|
|
|
|
it('calls the onSave callback when the state has changed', async function () {
|
|
let onSaveCalled = false;
|
|
|
|
const {result} = renderHook(() => useForm({
|
|
initialState: {a: 1},
|
|
onSave: () => {
|
|
onSaveCalled = true;
|
|
}
|
|
}));
|
|
|
|
act(() => result.current.updateForm(state => ({...state, a: 2})));
|
|
assert.equal(await act(() => result.current.handleSave()), true);
|
|
|
|
assert.equal(result.current.saveState, 'saved');
|
|
assert.equal(onSaveCalled, true);
|
|
});
|
|
});
|
|
});
|