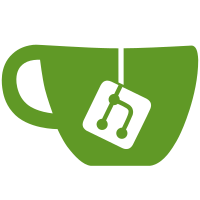
ref PROD-233 - Stored whether Docker is used in the config files - When running `yarn setup`, any existing Docker container will be reset. Run with `-y` to skip the confirmation step. - `yarn setup` will always init the database and generate fake data - Increased amount of default generated data to 100,000 members + 500 posts. - Made lots of performance improvements in the data generator so we can generate the default data in ±170s
32 lines
1.0 KiB
JavaScript
32 lines
1.0 KiB
JavaScript
const TableImporter = require('./TableImporter');
|
|
const {faker} = require('@faker-js/faker');
|
|
const {slugify} = require('@tryghost/string');
|
|
const security = require('@tryghost/security');
|
|
const dateToDatabaseString = require('../utils/database-date');
|
|
|
|
class UsersImporter extends TableImporter {
|
|
static table = 'users';
|
|
static dependencies = [];
|
|
defaultQuantity = 8;
|
|
|
|
constructor(knex, transaction) {
|
|
super(UsersImporter.table, knex, transaction);
|
|
}
|
|
|
|
async generate() {
|
|
const name = `${faker.name.firstName()} ${faker.name.lastName()}`;
|
|
return {
|
|
id: this.fastFakeObjectId(),
|
|
name: name,
|
|
slug: slugify(name),
|
|
password: await security.password.hash(faker.color.human()),
|
|
email: faker.internet.email(name),
|
|
profile_image: faker.internet.avatar(),
|
|
created_at: dateToDatabaseString(faker.date.between(new Date(2016, 0), new Date())),
|
|
created_by: 'unused'
|
|
};
|
|
}
|
|
}
|
|
|
|
module.exports = UsersImporter;
|