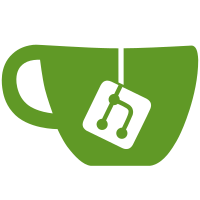
closes #10773 - The refactoring is a substitute for `urlService.utils` used previously throughout the codebase and now extracted into the separate module in Ghost-SDK - Added url-utils stubbing utility for test suites - Some tests had to be refactored to avoid double mocks (when url's are being reset inside of rested 'describe' groups)
51 lines
1.5 KiB
JavaScript
51 lines
1.5 KiB
JavaScript
const _ = require('lodash');
|
|
const sinon = require('sinon');
|
|
const UrlUtils = require('@tryghost/url-utils');
|
|
const config = require('../../server/config');
|
|
const urlUtils = require('../../server/lib/url-utils');
|
|
|
|
const defaultSandbox = sinon.createSandbox();
|
|
|
|
const getInstance = (options) => {
|
|
const opts = {
|
|
url: options.url,
|
|
adminUrl: options.adminUrl,
|
|
apiVersions: options.apiVersions,
|
|
slugs: options.slugs,
|
|
redirectCacheMaxAge: options.redirectCacheMaxAge,
|
|
baseApiPath: '/ghost/api'
|
|
};
|
|
|
|
return new UrlUtils(opts);
|
|
};
|
|
|
|
const stubUrlUtils = (options, sandbox) => {
|
|
const stubInstance = getInstance(options);
|
|
|
|
Object.keys(urlUtils).forEach((key) => {
|
|
sandbox.stub(urlUtils, key).callsFake(stubInstance[key]);
|
|
});
|
|
};
|
|
|
|
// Method for regressions tests must be used with restore method
|
|
const stubUrlUtilsFromConfig = () => {
|
|
const options = {
|
|
url: config.get('url'),
|
|
adminUrl: config.get('admin:url'),
|
|
apiVersions: config.get('api:versions'),
|
|
slugs: config.get('slugs').protected,
|
|
redirectCacheMaxAge: config.get('caching:301:maxAge'),
|
|
baseApiPath: '/ghost/api'
|
|
};
|
|
stubUrlUtils(options, defaultSandbox);
|
|
};
|
|
|
|
const restore = () => {
|
|
defaultSandbox.restore();
|
|
};
|
|
|
|
module.exports.stubUrlUtils = stubUrlUtils;
|
|
module.exports.stubUrlUtilsFromConfig = stubUrlUtilsFromConfig;
|
|
module.exports.restore = restore;
|
|
module.exports.getInstance = getInstance;
|