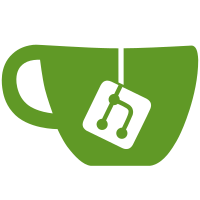
fix https://linear.app/tryghost/issue/SLO-155/paramsmap-is-not-a-function-an-unexpected-error-occurred-please-try - in the case you provide an object to the API, this code will throw an error because it can't map over an object - we can just assert that params should be an array and throw an error otherwise
33 lines
826 B
JavaScript
33 lines
826 B
JavaScript
const _ = require('lodash');
|
|
const {IncorrectUsageError} = require('@tryghost/errors');
|
|
|
|
/**
|
|
* @description Helper function to prepare params for internal usages.
|
|
*
|
|
* e.g. "a,B,c" -> ["a", "b", "c"]
|
|
*
|
|
* @param {String} params
|
|
* @return {Array}
|
|
*/
|
|
const trimAndLowerCase = (params) => {
|
|
params = params || '';
|
|
|
|
if (_.isString(params)) {
|
|
params = params.split(',');
|
|
}
|
|
|
|
// If we don't have an array at this point, something is wrong, so we should throw an
|
|
// error to avoid trying to .map over something else
|
|
if (!_.isArray(params)) {
|
|
throw new IncorrectUsageError({
|
|
message: 'Params must be a string or array'
|
|
});
|
|
}
|
|
|
|
return params.map((item) => {
|
|
return item.trim().toLowerCase();
|
|
});
|
|
};
|
|
|
|
module.exports.trimAndLowerCase = trimAndLowerCase;
|