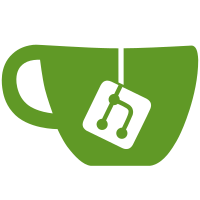
refs https://ghost.slack.com/archives/C02G9E68C/p1692784819620269 refs https://github.com/TryGhost/Product/issues/3504 - Somehow, when using i18n in TypeScript, the require will put some of the keys into 'default' and not into the root. Mainly all keys that have a space in them. Couldn't find any documentation about this - The solution is to also add 'default' to the keys that are being used in the code This change also fixes the translate script (wasn't updated for updated paths), includes the missing translations, and already adds comments to lookup translation strings
73 lines
2.6 KiB
JavaScript
73 lines
2.6 KiB
JavaScript
const assert = require('assert/strict');
|
|
const fs = require('fs/promises');
|
|
const path = require('path');
|
|
|
|
const i18n = require('../');
|
|
|
|
describe('i18n', function () {
|
|
it('does not have mismatched brackets in variables', async function () {
|
|
for (const locale of i18n.SUPPORTED_LOCALES) {
|
|
const translationFiles = await fs.readdir(path.join(`./locales/`, locale));
|
|
|
|
for (const file of translationFiles) {
|
|
const translationFile = require(path.join(`../locales/`, locale, file));
|
|
|
|
for (const key of Object.keys(translationFile)) {
|
|
const keyStartCount = key.match(/{{/g)?.length;
|
|
assert.equal(keyStartCount, key.match(/}}/g)?.length, `[${locale}/${file}] mismatched brackets in ${key}`);
|
|
|
|
const value = translationFile[key];
|
|
if (typeof value === 'string') {
|
|
const valueStartCount = value.match(/{{/g)?.length;
|
|
assert.equal(valueStartCount, value.match(/}}/g)?.length, `[${locale}/${file}] mismatched brackets in ${value}`);
|
|
|
|
// Maybe enable in the future if we want to enforce this
|
|
//if (value !== '') {
|
|
// assert.equal(keyStartCount, valueStartCount, `[${locale}/${file}] mismatched brackets between ${key} and ${value}`);
|
|
//}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
});
|
|
|
|
it('is uses default export if available', async function () {
|
|
const translationFile = require(path.join(`../locales/`, 'nl', 'portal.json'));
|
|
translationFile.Name = undefined;
|
|
translationFile.default = {
|
|
Name: 'Naam'
|
|
};
|
|
|
|
const t = i18n('nl', 'portal').t;
|
|
assert.equal(t('Name'), 'Naam');
|
|
});
|
|
|
|
describe('Can use Portal resources', function () {
|
|
describe('Dutch', function () {
|
|
let t;
|
|
|
|
before(function () {
|
|
t = i18n('nl', 'portal').t;
|
|
});
|
|
|
|
it('can translate `Name`', function () {
|
|
assert.equal(t('Name'), 'Naam');
|
|
});
|
|
});
|
|
});
|
|
|
|
describe('Can use Signup-form resources', function () {
|
|
describe('Afrikaans', function () {
|
|
let t;
|
|
|
|
before(function () {
|
|
t = i18n('af', 'signup-form').t;
|
|
});
|
|
|
|
it('can translate `Now check your email!`', function () {
|
|
assert.equal(t('Now check your email!'), 'Kyk nou in jou e-pos!');
|
|
});
|
|
});
|
|
});
|
|
});
|