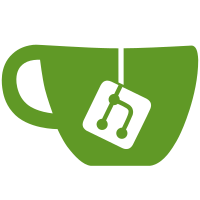
refs #9742, refs https://github.com/TryGhost/Ghost-CLI/issues/759 - required a reordering of Ghost's bootstrap file, because: - we have to ensure that no database queries are executed within Ghost during the migrations - make 3 sections: check if db needs initialisation, bootstrap Ghost with minimal components (db/models, express apps, load settings+theme) - create a new `migrator` utility, which tells you which state your db is in and offers an API to execute knex-migrator based on this state - ensure we still detect an incompatible db: you connect your 2.0 blog with a 0.11 database - enable maintenance mode if migrations are missing - if the migration have failed, knex-migrator roll auto rollback - you can automatically switch to 1.0 again - added socket communication for the CLI
73 lines
2.1 KiB
JavaScript
73 lines
2.1 KiB
JavaScript
const KnexMigrator = require('knex-migrator'),
|
|
config = require('../../config'),
|
|
common = require('../../lib/common'),
|
|
knexMigrator = new KnexMigrator({
|
|
knexMigratorFilePath: config.get('paths:appRoot')
|
|
});
|
|
|
|
module.exports.getState = () => {
|
|
let state, err;
|
|
|
|
return knexMigrator.isDatabaseOK()
|
|
.then(() => {
|
|
state = 1;
|
|
return state;
|
|
})
|
|
.catch((_err) => {
|
|
err = _err;
|
|
|
|
// CASE: database was never created
|
|
if (err.code === 'DB_NOT_INITIALISED') {
|
|
state = 2;
|
|
return state;
|
|
}
|
|
|
|
// CASE: you have created the database on your own, you have an existing none compatible db?
|
|
if (err.code === 'MIGRATION_TABLE_IS_MISSING') {
|
|
state = 3;
|
|
return state;
|
|
}
|
|
|
|
// CASE: database needs migrations
|
|
if (err.code === 'DB_NEEDS_MIGRATION') {
|
|
state = 4;
|
|
return state;
|
|
}
|
|
|
|
// CASE: database connection errors, unknown cases
|
|
throw err;
|
|
});
|
|
};
|
|
|
|
module.exports.dbInit = () => {
|
|
return knexMigrator.init();
|
|
};
|
|
|
|
module.exports.migrate = () => {
|
|
return knexMigrator.migrate();
|
|
};
|
|
|
|
module.exports.isDbCompatible = (connection) => {
|
|
return connection.raw('SELECT `key` FROM settings WHERE `key`="databaseVersion";')
|
|
.then((response) => {
|
|
if (!response || !response[0].length) {
|
|
return;
|
|
}
|
|
|
|
throw new common.errors.DatabaseVersionError({
|
|
message: 'Your database version is not compatible with Ghost 2.0.',
|
|
help: 'Want to keep your DB? Use Ghost < 1.0.0 or the "0.11" branch.' +
|
|
'\n\n\n' +
|
|
'Want to migrate Ghost 0.11 to 2.0? Please visit https://docs.ghost.org/v1/docs/migrating-to-ghost-1-0-0'
|
|
});
|
|
})
|
|
.catch((err) => {
|
|
// CASE settings table doesn't exists
|
|
if (err.errno === 1146 || err.errno === 1) {
|
|
return;
|
|
}
|
|
|
|
throw err;
|
|
});
|
|
};
|