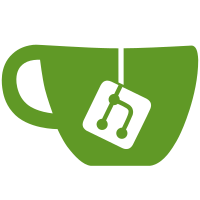
no issue The `settings` service has been a source of confusion when writing with modern Ember patterns because it's use of the deprecated `ProxyMixin` forced all property access/setting to go via `.get()` and `.set()` whereas the rest of the system has mostly (there are a few other uses of ProxyObjects remaining) eliminated the use of the non-native get/set methods. - removed use of `ProxyMixin` in the `settings` service by grabbing the attributes off the setting model after fetching and using `Object.defineProperty()` to add native getters/setters that pass through to the model's getters/setters. Ember's autotracking automatically works across the native getters/setters so we can then use the service as if it was any other native object - updated all code to use `settings.{attrName}` directly for getting/setting instead of `.get()` and `.set()` - removed use of observer in the `customViews` service because it was being set up before the native properties had been added on the settings service meaning autotracking wasn't able to set up properly
55 lines
1.6 KiB
JavaScript
55 lines
1.6 KiB
JavaScript
import Service, {inject as service} from '@ember/service';
|
|
import {action} from '@ember/object';
|
|
import {observes} from '@ember-decorators/object';
|
|
import {tracked} from '@glimmer/tracking';
|
|
|
|
const DEFAULT_SETTINGS = {
|
|
expanded: {
|
|
posts: true
|
|
}
|
|
};
|
|
|
|
export default class NavigationService extends Service {
|
|
@service session;
|
|
|
|
@tracked settings;
|
|
|
|
constructor() {
|
|
super(...arguments);
|
|
this.settings = Object.assign({}, DEFAULT_SETTINGS);
|
|
this.updateSettings();
|
|
}
|
|
|
|
// eslint-disable-next-line ghost/ember/no-observers
|
|
@observes('session.{isAuthenticated,user}', 'session.user.accessibility')
|
|
async updateSettings() {
|
|
// avoid fetching user before authenticated otherwise the 403 can fire
|
|
// during authentication and cause errors during setup/signin
|
|
if (!this.session.isAuthenticated || !this.session.user) {
|
|
return;
|
|
}
|
|
|
|
let userSettings = JSON.parse(this.session.user.accessibility || '{}') || {};
|
|
this.settings = userSettings.navigation || Object.assign({}, DEFAULT_SETTINGS);
|
|
}
|
|
|
|
@action
|
|
async toggleExpansion(key) {
|
|
if (!this.settings.expanded) {
|
|
this.settings.expanded = {};
|
|
}
|
|
|
|
this.settings.expanded[key] = !this.settings.expanded[key];
|
|
|
|
return await this._saveNavigationSettings();
|
|
}
|
|
|
|
async _saveNavigationSettings() {
|
|
let user = this.session.user;
|
|
let userSettings = JSON.parse(user.get('accessibility')) || {};
|
|
userSettings.navigation = this.settings;
|
|
user.set('accessibility', JSON.stringify(userSettings));
|
|
return user.save();
|
|
}
|
|
}
|