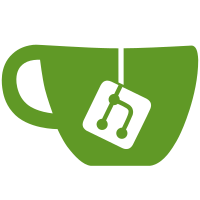
closes ENG-730 closes https://linear.app/tryghost/issue/ENG-730/ We've updated the input serializer to parse the filter, and responded with an error if it cannot be parsed correctly. Now that it's parsed, we can pass a mongo query object through the stack, which will lend itself to better typing for this code, which is a direction we want to go in anyway. We've had to update all the internal usages of the `browse` method to use mongo query objects.
67 lines
1.5 KiB
JavaScript
67 lines
1.5 KiB
JavaScript
const nql = require('@tryghost/nql');
|
|
|
|
/**
|
|
* @typedef {import('./Tier')} Tier
|
|
*/
|
|
|
|
class InMemoryTierRepository {
|
|
/** @type {Tier[]} */
|
|
#store = [];
|
|
/** @type {Object.<string, true>} */
|
|
#ids = {};
|
|
|
|
/**
|
|
* @param {Tier} tier
|
|
* @returns {any}
|
|
*/
|
|
toPrimitive(tier) {
|
|
return {
|
|
...tier,
|
|
active: (tier.status === 'active'),
|
|
type: tier.type,
|
|
id: tier.id.toHexString()
|
|
};
|
|
}
|
|
|
|
/**
|
|
* @param {Tier} linkClick
|
|
* @returns {Promise<void>}
|
|
*/
|
|
async save(tier) {
|
|
if (this.#ids[tier.id.toHexString()]) {
|
|
const existing = this.#store.findIndex((item) => {
|
|
return item.id.equals(tier.id);
|
|
});
|
|
this.#store.splice(existing, 1, tier);
|
|
} else {
|
|
this.#store.push(tier);
|
|
this.#ids[tier.id.toHexString()] = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param {import('bson-objectid').default} id
|
|
* @returns {Promise<Tier>}
|
|
*/
|
|
async getById(id) {
|
|
return this.#store.find((item) => {
|
|
return item.id.equals(id);
|
|
});
|
|
}
|
|
|
|
/**
|
|
* @param {object} [options]
|
|
* @param {string} [options.filter]
|
|
* @returns {Promise<Tier[]>}
|
|
*/
|
|
async getAll(options = {}) {
|
|
const filter = nql();
|
|
filter.filter = options.filter || {};
|
|
return this.#store.slice().filter((item) => {
|
|
return filter.queryJSON(this.toPrimitive(item));
|
|
});
|
|
}
|
|
}
|
|
|
|
module.exports = InMemoryTierRepository;
|