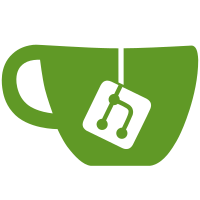
no issue - When Ghost is running in a test environment, it is configured with an invalid Stripe key that looks like `sk_test***`. In this case the migrations try runnig creating request to Stripe, which fail. The failures pollute the output, which makes other valid errors lost. - An example of such error log is following: ``` Invalid API Key provided: sk_test_******ripe ---------------------------------------- Error: Invalid API Key provided: sk_test_******ripe at res.toJSON.then.StripeAPIError.message (/home/naz/Workspace/Ghost/Ghost/node_modules/stripe/lib/StripeResource.js:214:23) at processTicksAndRejections (node:internal/process/task_queues:96:5) ``` - There doesn't seem to be a good reason to do migrations in the test environment. Skipping them as a special case to fix the output pollution problem seems like a right solution
162 lines
5.1 KiB
JavaScript
162 lines
5.1 KiB
JavaScript
const assert = require('assert');
|
|
const sinon = require('sinon');
|
|
const Migrations = require('../../../lib/Migrations');
|
|
|
|
describe('Migrations', function () {
|
|
describe('updateStripeProductNamesFromDefaultProduct', function () {
|
|
it('Does not run migration if api is not configured', async function () {
|
|
const api = {
|
|
getProduct: sinon.stub().resolves({
|
|
id: 'prod_123',
|
|
name: 'Bronze'
|
|
}),
|
|
_configured: false
|
|
};
|
|
const models = {
|
|
Product: {
|
|
transaction: sinon.spy()
|
|
}
|
|
};
|
|
|
|
const migrations = new Migrations({
|
|
models,
|
|
api
|
|
});
|
|
|
|
await migrations.execute();
|
|
|
|
assert(
|
|
models.Product.transaction.called === false,
|
|
'Stripe should not call any of the populateProductsAndPrices method parts if api is not configured'
|
|
);
|
|
});
|
|
|
|
it('Does not run migration if api is in test environment', async function () {
|
|
const api = {
|
|
getProduct: sinon.stub().resolves({
|
|
id: 'prod_123',
|
|
name: 'Bronze'
|
|
}),
|
|
_configured: true,
|
|
testEnv: true
|
|
};
|
|
const models = {
|
|
Product: {
|
|
transaction: sinon.spy()
|
|
}
|
|
};
|
|
|
|
const migrations = new Migrations({
|
|
models,
|
|
api
|
|
});
|
|
|
|
await migrations.execute();
|
|
|
|
assert(
|
|
models.Product.transaction.called === false,
|
|
'Stripe should not call any of the populateProductsAndPrices method parts if api is not configured'
|
|
);
|
|
});
|
|
|
|
it('Does not update Stripe product if name is not "Default Product"', async function () {
|
|
const api = {
|
|
getProduct: sinon.stub().resolves({
|
|
id: 'prod_123',
|
|
name: 'Bronze'
|
|
}),
|
|
updateProduct: sinon.stub().resolves()
|
|
};
|
|
const models = {
|
|
Product: {
|
|
transaction: fn => fn()
|
|
},
|
|
StripeProduct: {
|
|
findPage: sinon.stub().resolves({
|
|
data: [{
|
|
get(key) {
|
|
return key;
|
|
}
|
|
}],
|
|
meta: {}
|
|
})
|
|
},
|
|
Settings: {
|
|
findOne: sinon.stub().resolves({
|
|
key: 'title',
|
|
value: 'Site Title'
|
|
})
|
|
}
|
|
};
|
|
const migrations = new Migrations({
|
|
models,
|
|
api
|
|
});
|
|
|
|
await migrations.updateStripeProductNamesFromDefaultProduct();
|
|
|
|
assert(
|
|
api.updateProduct.called === false,
|
|
'Stripe product should not be updated if name is not "Default Product"'
|
|
);
|
|
});
|
|
|
|
it('Updates the Stripe Product name if it is Default Product', async function () {
|
|
const api = {
|
|
getProduct: sinon.stub().resolves({
|
|
id: 'prod_123',
|
|
name: 'Default Product'
|
|
}),
|
|
updateProduct: sinon.stub().resolves()
|
|
};
|
|
const models = {
|
|
Product: {
|
|
transaction: fn => fn()
|
|
},
|
|
StripeProduct: {
|
|
findPage: sinon.stub().resolves({
|
|
data: [{
|
|
get(key) {
|
|
return key;
|
|
}
|
|
}],
|
|
meta: {}
|
|
})
|
|
},
|
|
Settings: {
|
|
findOne: sinon.stub().resolves({
|
|
get(key) {
|
|
if (key === 'key') {
|
|
return 'title';
|
|
}
|
|
if (key === 'value') {
|
|
return 'Site Title';
|
|
}
|
|
|
|
return key;
|
|
}
|
|
})
|
|
}
|
|
};
|
|
const migrations = new Migrations({
|
|
models,
|
|
api
|
|
});
|
|
|
|
await migrations.updateStripeProductNamesFromDefaultProduct();
|
|
|
|
assert(
|
|
api.updateProduct.calledOnce,
|
|
'Stripe product should be updated if name is "Default Product"'
|
|
);
|
|
|
|
assert(
|
|
api.updateProduct.calledWith('prod_123', {
|
|
name: 'Site Title'
|
|
}),
|
|
'Stripe product should have been updated with the site title as name'
|
|
);
|
|
});
|
|
});
|
|
});
|