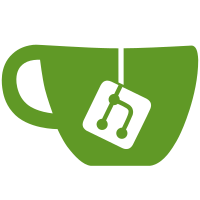
refs https://github.com/TryGhost/Team/issues/3168 - This is basic scaffolding for collection resources UI in Admin. For the most part it's a copy-paste of tags code with slight modifications to fit the collections usecase
39 lines
1009 B
JavaScript
39 lines
1009 B
JavaScript
import Controller from '@ember/controller';
|
|
import {action} from '@ember/object';
|
|
import {inject as service} from '@ember/service';
|
|
import {tracked} from '@glimmer/tracking';
|
|
|
|
export default class CollectionsController extends Controller {
|
|
@service router;
|
|
|
|
queryParams = ['type'];
|
|
@tracked type = 'public';
|
|
|
|
get collections() {
|
|
return this.model;
|
|
}
|
|
|
|
get filteredCollections() {
|
|
return this.collections.filter((collection) => {
|
|
return (!collection.isNew);
|
|
});
|
|
}
|
|
|
|
get sortedCollections() {
|
|
return this.filteredCollections.sort((collectionA, collectionB) => {
|
|
// ignorePunctuation means the # in internal collection names is ignored
|
|
return collectionA.title.localeCompare(collectionB.title, undefined, {ignorePunctuation: true});
|
|
});
|
|
}
|
|
|
|
@action
|
|
changeType(type) {
|
|
this.type = type;
|
|
}
|
|
|
|
@action
|
|
newCollection() {
|
|
this.router.transitionTo('collection.new');
|
|
}
|
|
}
|