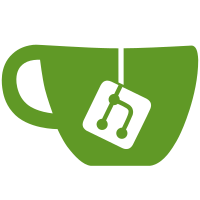
refs https://github.com/TryGhost/Team/issues/1141 Showing canceled subscriptions provide a more complete picture of the activity of a member. - Given there is no `member.product` object when a subscription is canceled, use the `member.subscriptions.price.product` objects instead of `member.products`. - applied boy-scout rule for linter errors and and code formatting - removed `multipleTiers` flag conditionals as it's now GA - set up subscriptions as a separate mirage resource so they are easier to work with - updated `PUT /members/:id/` endpoint to match real API's complimentary subscription behaviour - modified mirage member serializer to match API output Co-authored-by: Kevin Ansfield <kevin@lookingsideways.co.uk> Co-authored-by: Peter Zimon <peter.zimon@gmail.com>
34 lines
1006 B
JavaScript
34 lines
1006 B
JavaScript
import BaseSerializer from './application';
|
|
|
|
export default BaseSerializer.extend({
|
|
embed: true,
|
|
|
|
include(request) {
|
|
let queryIncludes = (request.queryParams.include || '').split(',').compact();
|
|
const includes = new Set(queryIncludes);
|
|
|
|
// embedded records that are included by default in the API
|
|
includes.add('labels');
|
|
includes.add('subscriptions');
|
|
|
|
return Array.from(includes);
|
|
},
|
|
|
|
serialize() {
|
|
const serialized = BaseSerializer.prototype.serialize.call(this, ...arguments);
|
|
|
|
// comped subscriptions are returned with a blank ID
|
|
// (we use `.comped` internally because mirage resources require an ID)
|
|
(serialized.members || [serialized.member]).forEach((member) => {
|
|
member.subscriptions.forEach((sub) => {
|
|
if (sub.comped) {
|
|
sub.id = '';
|
|
}
|
|
delete sub.comped;
|
|
});
|
|
});
|
|
|
|
return serialized;
|
|
}
|
|
});
|