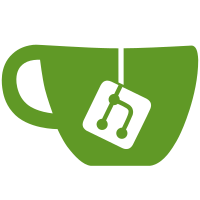
refs https://github.com/TryGhost/Team/issues/2077 - The "productRepository" methods have been deprecated in favor of "tiers" and "Tiers API". - The changes migrated usages of "productRepository.getDefaultProduct" to Tiers API's "readDefaultTier"
82 lines
2.1 KiB
JavaScript
82 lines
2.1 KiB
JavaScript
const assert = require('assert');
|
|
const TiersAPI = require('../lib/TiersAPI');
|
|
const InMemoryTierRepository = require('../lib/InMemoryTierRepository');
|
|
|
|
describe('TiersAPI', function () {
|
|
/** @type {TiersAPI.ITierRepository} */
|
|
let repository;
|
|
|
|
/** @type {TiersAPI} */
|
|
let api;
|
|
|
|
before(function () {
|
|
repository = new InMemoryTierRepository();
|
|
api = new TiersAPI({
|
|
repository,
|
|
slugService: {
|
|
async generate(input) {
|
|
return input;
|
|
}
|
|
}
|
|
});
|
|
});
|
|
|
|
it('Can not create new free Tiers', async function () {
|
|
let error;
|
|
try {
|
|
await api.add({
|
|
name: 'My testing Tier',
|
|
type: 'free'
|
|
});
|
|
error = null;
|
|
} catch (err) {
|
|
error = err;
|
|
} finally {
|
|
assert(error, 'An error should have been thrown');
|
|
}
|
|
});
|
|
|
|
it('Can create new paid Tiers and find them again', async function () {
|
|
const tier = await api.add({
|
|
name: 'My testing Tier',
|
|
type: 'paid',
|
|
monthlyPrice: 5000,
|
|
yearlyPrice: 50000,
|
|
currency: 'usd'
|
|
});
|
|
|
|
const found = await api.read(tier.id.toHexString());
|
|
|
|
assert(found);
|
|
});
|
|
|
|
it('Can edit a tier', async function () {
|
|
const tier = await api.add({
|
|
name: 'My testing Tier',
|
|
type: 'paid',
|
|
monthlyPrice: 5000,
|
|
yearlyPrice: 50000,
|
|
currency: 'usd'
|
|
});
|
|
|
|
const updated = await api.edit(tier.id.toHexString(), {
|
|
name: 'Updated'
|
|
});
|
|
|
|
assert(updated.name === 'Updated');
|
|
});
|
|
|
|
it('Can browse tiers', async function () {
|
|
const page = await api.browse();
|
|
|
|
assert(page.data.length === 2);
|
|
assert(page.meta.pagination.total === 2);
|
|
});
|
|
|
|
it('Can read a default tier', async function () {
|
|
const defaultTier = await api.readDefaultTier();
|
|
|
|
assert.equal(defaultTier?.name, 'My testing Tier');
|
|
});
|
|
});
|