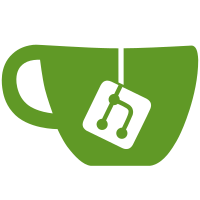
no issue Reduces likelihood of seeing the "Loading editor..." state when accessing the editor by fetching the editor module in the background after login. - added `koenig` service - `.resource` getter for returning a React suspense resource object to reduce duplication across main editor and HTML input components - `.fetch()` method that fetches the Koenig module, avoids concurrent or repeated fetches by utilising an internal `._fetchTask` ember-concurrency task - updated Koenig components to use the `koenig` service and associated resource for fetching the module - required setting up `editorResource` inside the component's class and passing it in as a prop to the suspense-aware components as we need access to Ember's dependency injection available in the class - added `koenig.fetch()` call to our post-login code in the `session` service so the fetch is started before the editor is accessed
55 lines
1.3 KiB
JavaScript
55 lines
1.3 KiB
JavaScript
import Service from '@ember/service';
|
|
import fetchKoenigLexical from '../utils/fetch-koenig-lexical';
|
|
import {task} from 'ember-concurrency';
|
|
|
|
export default class Koenig extends Service {
|
|
get resource() {
|
|
let status = 'pending';
|
|
let response;
|
|
|
|
const suspender = this.fetch().then(
|
|
(res) => {
|
|
status = 'success';
|
|
response = res;
|
|
},
|
|
(err) => {
|
|
status = 'error';
|
|
response = err;
|
|
}
|
|
);
|
|
|
|
const read = () => {
|
|
switch (status) {
|
|
case 'pending':
|
|
throw suspender;
|
|
case 'error':
|
|
throw response;
|
|
default:
|
|
return response;
|
|
}
|
|
};
|
|
|
|
return {read};
|
|
}
|
|
|
|
async fetch() {
|
|
// avoid re-fetching whilst already fetching
|
|
if (this._fetchTask.isRunning) {
|
|
return await this._fetchTask.last;
|
|
}
|
|
|
|
// avoid re-fetching if we've already fetched successfully
|
|
if (this._fetchTask.lastSuccessful) {
|
|
return this._fetchTask.lastSuccessful.value;
|
|
}
|
|
|
|
// kick-off a new fetch
|
|
return await this._fetchTask.perform();
|
|
}
|
|
|
|
@task
|
|
*_fetchTask() {
|
|
return yield fetchKoenigLexical();
|
|
}
|
|
}
|