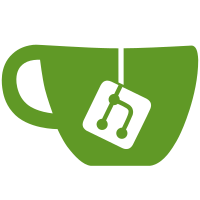
refs https://github.com/TryGhost/Team/issues/1057 This method will validate a token, and then return the member associated with it. Rather than exposing token validation and coupling consumers to the structure of the token response data.
54 lines
1.3 KiB
JavaScript
54 lines
1.3 KiB
JavaScript
const jose = require('node-jose');
|
|
const jwt = require('jsonwebtoken');
|
|
|
|
module.exports = class TokenService {
|
|
constructor({
|
|
privateKey,
|
|
publicKey,
|
|
issuer
|
|
}) {
|
|
this._keyStore = jose.JWK.createKeyStore();
|
|
this._keyStoreReady = this._keyStore.add(privateKey, 'pem');
|
|
this._privateKey = privateKey;
|
|
this._publicKey = publicKey;
|
|
this._issuer = issuer;
|
|
}
|
|
|
|
async encodeIdentityToken({sub}) {
|
|
const jwk = await this._keyStoreReady;
|
|
return jwt.sign({
|
|
sub,
|
|
kid: jwk.kid
|
|
}, this._privateKey, {
|
|
algorithm: 'RS512',
|
|
audience: this._issuer,
|
|
expiresIn: '10m',
|
|
issuer: this._issuer
|
|
});
|
|
}
|
|
|
|
/**
|
|
* @param {string} token
|
|
* @returns {Promise<jwt.JwtPayload>}
|
|
*/
|
|
async decodeToken(token) {
|
|
await this._keyStoreReady;
|
|
|
|
const result = jwt.verify(token, this._publicKey, {
|
|
algorithms: ['RS512'],
|
|
issuer: this._issuer
|
|
});
|
|
|
|
if (typeof result === 'string') {
|
|
return {sub: result};
|
|
}
|
|
|
|
return result;
|
|
}
|
|
|
|
async getPublicKeys() {
|
|
await this._keyStoreReady;
|
|
return this._keyStore.toJSON();
|
|
}
|
|
};
|