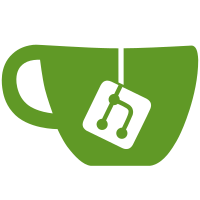
no issue - we don't want accidental background clicks closing this modal as it contains complex UI rather than a simple notification - the members import modal is still using the old/outdated modal pattern so there was no option for disabling background click, went with a quick-fix for now rather than updating everything to our modern modal patterns - added passthrough of arguments to the `close` action on `<GhFullscreenModal>` - updated `<GhFullscreenModal>` background click handler to pass "background" as an action argument - updated the action used for handling members import modal closing to skip closing when it receives "background" as the first argument
61 lines
1.5 KiB
JavaScript
61 lines
1.5 KiB
JavaScript
import Component from '@ember/component';
|
|
import RSVP from 'rsvp';
|
|
import {computed} from '@ember/object';
|
|
import {A as emberA} from '@ember/array';
|
|
import {isBlank} from '@ember/utils';
|
|
import {run} from '@ember/runloop';
|
|
import {inject as service} from '@ember/service';
|
|
|
|
const FullScreenModalComponent = Component.extend({
|
|
dropdown: service(),
|
|
|
|
model: null,
|
|
modifier: null,
|
|
|
|
modalPath: computed('modal', function () {
|
|
return `modal-${this.modal || 'unknown'}`;
|
|
}),
|
|
|
|
modalClasses: computed('modifier', function () {
|
|
let modalClass = 'fullscreen-modal';
|
|
let modifiers = (this.modifier || '').split(' ');
|
|
let modalClasses = emberA([modalClass]);
|
|
|
|
modifiers.forEach((modifier) => {
|
|
if (!isBlank(modifier)) {
|
|
let className = `${modalClass}-${modifier}`;
|
|
modalClasses.push(className);
|
|
}
|
|
});
|
|
|
|
return modalClasses.join(' ');
|
|
}),
|
|
|
|
didInsertElement() {
|
|
this._super(...arguments);
|
|
run.schedule('afterRender', this, function () {
|
|
this.dropdown.closeDropdowns();
|
|
});
|
|
},
|
|
|
|
actions: {
|
|
close() {
|
|
return this.close(...arguments);
|
|
},
|
|
|
|
confirm() {
|
|
return this.confirm(...arguments);
|
|
},
|
|
|
|
clickOverlay() {
|
|
this.send('close', ...arguments);
|
|
}
|
|
},
|
|
|
|
// Allowed actions
|
|
close: () => RSVP.resolve(),
|
|
confirm: () => RSVP.resolve()
|
|
});
|
|
|
|
export default FullScreenModalComponent;
|