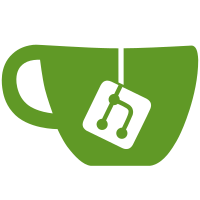
issue https://github.com/TryGhost/Team/issues/857 - The goal is to avoid testing for the owner role only is cases where we should be testing for the owner or admin role - `isOwner` => `isOwnerOnly` - `isAdmin` => `isAdminOnly` - `isOwnerOrAdmin` => `isAdmin` (concerns now both Owner and Admins)
39 lines
1.2 KiB
JavaScript
39 lines
1.2 KiB
JavaScript
import {describe, it} from 'mocha';
|
|
import {expect} from 'chai';
|
|
import {ghUserCanAdmin} from 'ghost-admin/helpers/gh-user-can-admin';
|
|
|
|
describe('Unit: Helper: gh-user-can-admin', function () {
|
|
// Mock up roles and test for truthy
|
|
describe('Owner or admin roles', function () {
|
|
let user = {
|
|
get(role) {
|
|
if (role === 'isAdmin') {
|
|
return true;
|
|
}
|
|
throw new Error('unsupported'); // Make sure we only call get('isAdmin')
|
|
}
|
|
};
|
|
|
|
it(' - can be Admin', function () {
|
|
let result = ghUserCanAdmin([user]);
|
|
expect(result).to.equal(true);
|
|
});
|
|
});
|
|
|
|
describe('Editor, Author & Contributor roles', function () {
|
|
let user = {
|
|
get(role) {
|
|
if (role === 'isAdmin') {
|
|
return false;
|
|
}
|
|
throw new Error('unsupported'); // Make sure we only call get('isAdmin')
|
|
}
|
|
};
|
|
|
|
it(' - cannot be Admin', function () {
|
|
let result = ghUserCanAdmin([user]);
|
|
expect(result).to.equal(false);
|
|
});
|
|
});
|
|
});
|