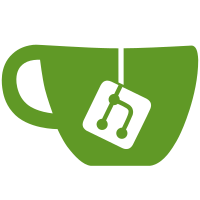
refs: https://github.com/TryGhost/DevOps/issues/11 This is a pretty huge commit, but the relevant points are: * Each importer no longer needs to be passed a set of data, it just gets the data it needs * Each importer specifies its dependencies, so that the order of import can be determined at runtime using a topological sort * The main data generator function can just tell each importer to import the data it has This makes working on the data generator much easier. Some other benefits are: * Batched importing, massively speeding up the whole process * `--tables` to set the exact tables you want to import, and specify the quantity of each
34 lines
1010 B
JavaScript
34 lines
1010 B
JavaScript
/**
|
|
* This sorting algorithm is used to make sure that dependent tables are imported after their dependencies.
|
|
* @param {Array<Object>} objects Objects with a name and dependencies properties
|
|
* @returns Topologically sorted array of objects
|
|
*/
|
|
module.exports = function topologicalSort(objects) {
|
|
// Create an empty result array to store the ordered objects
|
|
const result = [];
|
|
// Create a set to track visited objects during the DFS
|
|
const visited = new Set();
|
|
|
|
// Helper function to perform DFS
|
|
function dfs(name) {
|
|
if (visited.has(name)) {
|
|
return;
|
|
}
|
|
|
|
visited.add(name);
|
|
const dependencies = objects.find(item => item.name === name)?.dependencies || [];
|
|
for (const dependency of dependencies) {
|
|
dfs(dependency);
|
|
}
|
|
|
|
result.push(objects.find(item => item.name === name));
|
|
}
|
|
|
|
// Perform DFS on each object
|
|
for (const object of objects) {
|
|
dfs(object.name);
|
|
}
|
|
|
|
return result;
|
|
};
|