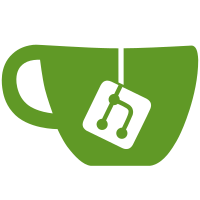
refs https://github.com/TryGhost/Team/issues/1054 This package will be used as a singleton for listenig and sending events throughout the members application.
50 lines
1.1 KiB
JavaScript
50 lines
1.1 KiB
JavaScript
const should = require('should');
|
|
const DomainEvents = require('../');
|
|
|
|
class TestEvent {
|
|
/**
|
|
* @param {string} message
|
|
*/
|
|
constructor(message) {
|
|
this.timestamp = new Date();
|
|
this.data = {
|
|
message
|
|
};
|
|
}
|
|
}
|
|
|
|
describe('DomainEvents', function () {
|
|
it('Will call multiple subscribers with the event when it is dispatched', function (done) {
|
|
const event = new TestEvent('Hello, world!');
|
|
|
|
let called = 0;
|
|
|
|
/**
|
|
* @param {TestEvent} receivedEvent
|
|
*/
|
|
function handler1(receivedEvent) {
|
|
should.equal(receivedEvent, event);
|
|
called += 1;
|
|
if (called === 2) {
|
|
done();
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param {TestEvent} receivedEvent
|
|
*/
|
|
function handler2(receivedEvent) {
|
|
should.equal(receivedEvent, event);
|
|
called += 1;
|
|
if (called === 2) {
|
|
done();
|
|
}
|
|
}
|
|
|
|
DomainEvents.subscribe(TestEvent, handler1);
|
|
DomainEvents.subscribe(TestEvent, handler2);
|
|
|
|
DomainEvents.dispatch(event);
|
|
});
|
|
});
|