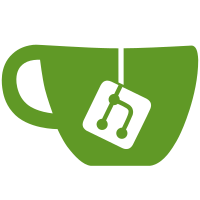
refs https://github.com/TryGhost/Team/issues/584 refs https://github.com/TryGhost/Team/issues/1498 - updated newsletter save routine in `edit-newsletter` modal to open an email confirmation modal if the API indicates one was sent - modal indicates that the previously set or default email will continue to be used until verified - response from API when saving looks like `{newsletters: [{...}], meta: {sent_email_verification: ['sender_name]}}` - added custom newsletter serializer and updated model so that the `meta` property returned in the response when saving posts is exposed - Ember Data only exposes meta on array-response find/query methods - https://github.com/emberjs/data/issues/2905 - added `/settings/members-email-labs/?verifyEmail=xyz` query param handling - opens email verification modal if param is set and instantly clears the query param to avoid problems with sticky params - when the modal opens it makes a `PUT /newsletters/verify-email/` request with the token in the body params, on the API side this works the same as a newsletter update request returning the fully updated newsletter record which is then pushed into the store - removed unused from/reply address code from `<Settings::MembersEmailLabs>` component and controller - setting the values now handled per-newsletter in the edit-newsletter modal - verifying email change is handled in the members-email-labs controller - fixed mirage not outputting pluralized root for "singular" endpoints such as POST/PUT requests to better match our API behaviour
51 lines
1.5 KiB
JavaScript
51 lines
1.5 KiB
JavaScript
import {Collection, RestSerializer} from 'miragejs';
|
|
import {pluralize} from 'ember-inflector';
|
|
import {underscore} from '@ember/string';
|
|
|
|
export default RestSerializer.extend({
|
|
keyForCollection(collection) {
|
|
return underscore(pluralize(collection));
|
|
},
|
|
|
|
keyForAttribute(attr) {
|
|
return underscore(attr);
|
|
},
|
|
|
|
keyForRelationship(relationship) {
|
|
return underscore(relationship);
|
|
},
|
|
|
|
keyForEmbeddedRelationship(relationship) {
|
|
return underscore(relationship);
|
|
},
|
|
|
|
keyForForeignKey(relationshipName) {
|
|
return `${underscore(relationshipName)}_id`;
|
|
},
|
|
|
|
serialize(object, request) {
|
|
if (this.isModel(object)) {
|
|
object = new Collection(object.modelName, [object]);
|
|
}
|
|
|
|
let json = RestSerializer.prototype.serialize.call(this, object, request);
|
|
|
|
if (this.isCollection(object) && object.meta) {
|
|
json.meta = object.meta;
|
|
}
|
|
|
|
return json;
|
|
},
|
|
|
|
// POST and PUT request send data in pluralized attributes for all models,
|
|
// so we extract it here - this allows #normalizedRequestAttrs to work
|
|
// in route functions
|
|
normalize(body, modelName) {
|
|
// sometimes mirage doesn't include a modelName, so we extrapolate it from
|
|
// the first element of Object.keys
|
|
modelName = pluralize(modelName) || Object.keys(body)[0];
|
|
let [attributes] = body[modelName] || [{}];
|
|
return {data: {attributes}};
|
|
}
|
|
});
|