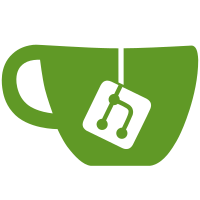
refs bd6a295674
- earlier this week I refactored this block of code to get rid of
explicit `stat` calls, to make the fs operations a little more
lightweight
- I inadvertantly forgot that readdir doesn't follow symlinks, and we
were previously use stat that does, so it was ignoring themes that were
symlinked into `content/themes`
- instead of rolling back my change, I've added an if-statement to call
`fs.stat` and check the origin of the symlink to see if it's a
directory
- also added a test that fails without this change
303 lines
11 KiB
JavaScript
303 lines
11 KiB
JavaScript
require('./utils');
|
|
|
|
const tmp = require('tmp');
|
|
const join = require('path').join;
|
|
const fs = require('fs-extra');
|
|
const packageJSON = require('../');
|
|
|
|
describe('package-json read', function () {
|
|
describe('readPackages', function () {
|
|
it('should read directory and ignore unneeded items', function (done) {
|
|
const packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'casper'));
|
|
fs.writeFileSync(join(packagePath.name, 'casper', 'index.hbs'), '');
|
|
|
|
// create some trash
|
|
fs.mkdirSync(join(packagePath.name, 'node_modules'));
|
|
fs.mkdirSync(join(packagePath.name, 'bower_components'));
|
|
fs.mkdirSync(join(packagePath.name, '.git'));
|
|
fs.writeFileSync(join(packagePath.name, '.DS_Store'), '');
|
|
|
|
packageJSON.readPackages(packagePath.name)
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
casper: {
|
|
name: 'casper',
|
|
path: join(packagePath.name, 'casper'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and parse package.json files', function (done) {
|
|
let packagePath;
|
|
let pkgJson;
|
|
|
|
packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
pkgJson = JSON.stringify({
|
|
name: 'test',
|
|
version: '0.0.0'
|
|
});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'testtheme'));
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'package.json'), pkgJson);
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'index.hbs'), '');
|
|
|
|
packageJSON.readPackages(packagePath.name)
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
testtheme: {
|
|
name: 'testtheme',
|
|
path: join(packagePath.name, 'testtheme'),
|
|
'package.json': {
|
|
name: 'test',
|
|
version: '0.0.0'
|
|
}
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and ignore invalid package.json files', function (done) {
|
|
let packagePath;
|
|
let pkgJson;
|
|
|
|
packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
pkgJson = JSON.stringify({
|
|
name: 'test'
|
|
});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'testtheme'));
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'package.json'), pkgJson);
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'index.hbs'), '');
|
|
|
|
packageJSON.readPackages(packagePath.name)
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
testtheme: {
|
|
name: 'testtheme',
|
|
path: join(packagePath.name, 'testtheme'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and include symlinked directories', function (done) {
|
|
let packagePath;
|
|
let pkgJson;
|
|
|
|
packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
pkgJson = JSON.stringify({
|
|
name: 'test'
|
|
});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'testtheme'));
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'package.json'), pkgJson);
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'index.hbs'), '');
|
|
|
|
// Symlink one theme to the other so we should have 2 themes
|
|
fs.symlinkSync(join(packagePath.name, 'testtheme'), join(packagePath.name, 'testtheme2'));
|
|
|
|
packageJSON.readPackages(packagePath.name)
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
testtheme: {
|
|
name: 'testtheme',
|
|
path: join(packagePath.name, 'testtheme'),
|
|
'package.json': null
|
|
},
|
|
testtheme2: {
|
|
name: 'testtheme2',
|
|
path: join(packagePath.name, 'testtheme2'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
});
|
|
|
|
describe('readPackage', function () {
|
|
it('should read directory and ignore unneeded items', function (done) {
|
|
const packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'casper'));
|
|
fs.writeFileSync(join(packagePath.name, 'casper', 'index.hbs'), '');
|
|
|
|
// create some trash
|
|
fs.mkdirSync(join(packagePath.name, 'node_modules'));
|
|
fs.mkdirSync(join(packagePath.name, 'bower_components'));
|
|
fs.mkdirSync(join(packagePath.name, '.git'));
|
|
fs.writeFileSync(join(packagePath.name, '.DS_Store'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'casper')
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
casper: {
|
|
name: 'casper',
|
|
path: join(packagePath.name, 'casper'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and parse package.json files', function (done) {
|
|
let packagePath;
|
|
let pkgJson;
|
|
|
|
packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
pkgJson = JSON.stringify({
|
|
name: 'test',
|
|
version: '0.0.0'
|
|
});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'testtheme'));
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'package.json'), pkgJson);
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'index.hbs'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'testtheme')
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
testtheme: {
|
|
name: 'testtheme',
|
|
path: join(packagePath.name, 'testtheme'),
|
|
'package.json': {
|
|
name: 'test',
|
|
version: '0.0.0'
|
|
}
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and ignore invalid package.json files', function (done) {
|
|
let packagePath;
|
|
let pkgJson;
|
|
|
|
packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
pkgJson = JSON.stringify({
|
|
name: 'test'
|
|
});
|
|
|
|
// create example theme
|
|
fs.mkdirSync(join(packagePath.name, 'testtheme'));
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'package.json'), pkgJson);
|
|
fs.writeFileSync(join(packagePath.name, 'testtheme', 'index.hbs'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'testtheme')
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
testtheme: {
|
|
name: 'testtheme',
|
|
path: join(packagePath.name, 'testtheme'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should read directory and include only single requested package', function (done) {
|
|
const packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
|
|
// create trash
|
|
fs.writeFileSync(join(packagePath.name, 'casper.zip'), '');
|
|
fs.writeFileSync(join(packagePath.name, '.DS_Store'), '');
|
|
|
|
// create actual theme
|
|
fs.mkdirSync(join(packagePath.name, 'casper'));
|
|
fs.mkdirSync(join(packagePath.name, 'casper', 'partials'));
|
|
fs.writeFileSync(join(packagePath.name, 'casper', 'index.hbs'), '');
|
|
fs.writeFileSync(join(packagePath.name, 'casper', 'partials', 'navigation.hbs'), '');
|
|
fs.mkdirSync(join(packagePath.name, 'not-casper'));
|
|
fs.writeFileSync(join(packagePath.name, 'not-casper', 'index.hbs'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'casper')
|
|
.then(function (pkgs) {
|
|
pkgs.should.eql({
|
|
casper: {
|
|
name: 'casper',
|
|
path: join(packagePath.name, 'casper'),
|
|
'package.json': null
|
|
}
|
|
});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should return an error if package cannot be found', function (done) {
|
|
const packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
|
|
// create trash
|
|
fs.writeFileSync(join(packagePath.name, 'casper.zip'), '');
|
|
fs.writeFileSync(join(packagePath.name, '.DS_Store'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'casper')
|
|
.then(function () {
|
|
done('Should have thrown an error');
|
|
})
|
|
.catch(function (err) {
|
|
err.message.should.eql('Package not found');
|
|
done();
|
|
})
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
|
|
it('should return empty object if package is not a directory', function (done) {
|
|
const packagePath = tmp.dirSync({unsafeCleanup: true});
|
|
|
|
// create trash
|
|
fs.writeFileSync(join(packagePath.name, 'casper.zip'), '');
|
|
fs.writeFileSync(join(packagePath.name, '.DS_Store'), '');
|
|
|
|
packageJSON.readPackage(packagePath.name, 'casper.zip')
|
|
.then(function (pkg) {
|
|
pkg.should.eql({});
|
|
|
|
done();
|
|
})
|
|
.catch(done)
|
|
.finally(packagePath.removeCallback);
|
|
});
|
|
});
|
|
});
|