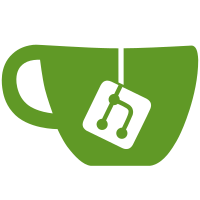
no issue Only Admins/Owners can browse members to get member counts but Editors/Authors are allowed to send email. This means we need to account for the count figures being missing. - added guard to the total member count fetch in `PublishOptions` - guard needed because the failed API request would abort setup - when the current user isn't an admin, set the total member count to 1 to avoid email options being disabled - added guard in the `{{members-count-fetcher}}` resource so we're not triggering API errors and the count is kept as `null` so it's handled automatically if passed to `{{gh-pluralize}}` - updated `<GhMembersRecipientSelect>` to not show counts (or the surrounding `()`) when the count is `null`
48 lines
1.4 KiB
JavaScript
48 lines
1.4 KiB
JavaScript
import {Resource} from 'ember-could-get-used-to-this';
|
|
import {inject as service} from '@ember/service';
|
|
import {task} from 'ember-concurrency';
|
|
import {tracked} from '@glimmer/tracking';
|
|
|
|
export default class MembersCount extends Resource {
|
|
@service membersCountCache;
|
|
@service session;
|
|
|
|
@tracked count = null;
|
|
|
|
get value() {
|
|
return {
|
|
isLoading: this.fetchMembersTask.isRunning,
|
|
count: this.count
|
|
};
|
|
}
|
|
|
|
setup() {
|
|
const query = this.args.named.query || {};
|
|
this._query = query;
|
|
this.fetchMembersTask.perform({query});
|
|
}
|
|
|
|
update() {
|
|
// required due to a weird invalidation issue when using Ember Data with ember-could-get-used-to-this
|
|
// TODO: re-test after upgrading to ember-resources
|
|
if (this.args.named.query !== this._query) {
|
|
const query = this.args.named.query || {};
|
|
this._query = query;
|
|
this.fetchMembersTask.perform({query});
|
|
}
|
|
}
|
|
|
|
@task
|
|
*fetchMembersTask({query} = {}) {
|
|
// Only Admins/Owners have access to the /members/ endpoint to fetch a count.
|
|
// For other roles simply leave it as `null` so templates can react accordingly
|
|
if (!this.session.user.isAdmin) {
|
|
this.count = null;
|
|
return;
|
|
}
|
|
|
|
const count = yield this.membersCountCache.count(query);
|
|
this.count = count;
|
|
}
|
|
}
|