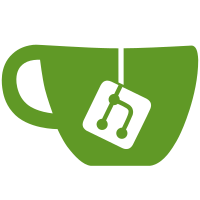
- This is a first pass at getting a more logical structure. The focus is on moving from admin/frontend to client/server. - The location of the databases is highly important, this isn't expected to change again In the future - client/assets should probably become public/ - more stuff should be shared (helpers etc) - cleanup some confusion around tpl and views
76 lines
2.0 KiB
JavaScript
76 lines
2.0 KiB
JavaScript
/*global window, document, Ghost, $, _, Backbone, JST */
|
|
(function () {
|
|
"use strict";
|
|
|
|
Ghost.View = Backbone.View.extend({
|
|
|
|
// Adds a subview to the current view, which will
|
|
// ensure its removal when this view is removed,
|
|
// or when view.removeSubviews is called
|
|
addSubview: function (view) {
|
|
if (!(view instanceof Backbone.View)) {
|
|
throw new Error("Subview must be a Backbone.View");
|
|
}
|
|
this.subviews = this.subviews || [];
|
|
this.subviews.push(view);
|
|
return view;
|
|
},
|
|
|
|
// Removes any subviews associated with this view
|
|
// by `addSubview`, which will in-turn remove any
|
|
// children of those views, and so on.
|
|
removeSubviews: function () {
|
|
var i, l, children = this.subviews;
|
|
if (!children) {
|
|
return this;
|
|
}
|
|
for (i = 0, l = children.length; i < l; i += 1) {
|
|
children[i].remove();
|
|
}
|
|
this.subviews = [];
|
|
return this;
|
|
},
|
|
|
|
// Extends the view's remove, by calling `removeSubviews`
|
|
// if any subviews exist.
|
|
remove: function () {
|
|
if (this.subviews) {
|
|
this.removeSubviews();
|
|
}
|
|
return Backbone.View.prototype.remove.apply(this, arguments);
|
|
}
|
|
|
|
});
|
|
|
|
Ghost.TemplateView = Ghost.View.extend({
|
|
templateName: "widget",
|
|
|
|
template: function (data) {
|
|
return JST[this.templateName](data);
|
|
},
|
|
|
|
templateData: function () {
|
|
if (this.model) {
|
|
return this.model.toJSON();
|
|
}
|
|
|
|
if (this.collection) {
|
|
return this.collection.toJSON();
|
|
}
|
|
|
|
return {};
|
|
},
|
|
|
|
render: function () {
|
|
this.$el.html(this.template(this.templateData()));
|
|
|
|
if (_.isFunction(this.afterRender)) {
|
|
this.afterRender();
|
|
}
|
|
|
|
return this;
|
|
}
|
|
});
|
|
|
|
}());
|