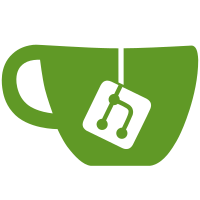
refs https://linear.app/tryghost/issue/CORE-84/have-a-look-at-the-eggs-redirects-refactor-branch
- In most of the packages we follow the pattern of passing in a single "options" object into a constructor and desructuring those the object into parameter, like this example: 077c83dc2d/packages/limit-service/lib/limit-service.js (L19-L26)
80 lines
2.2 KiB
JavaScript
80 lines
2.2 KiB
JavaScript
const should = require('should');
|
|
const DynamicRedirectManager = require('../');
|
|
|
|
const urlUtils = {
|
|
getSubdir() {
|
|
return '';
|
|
},
|
|
urlJoin(...parts) {
|
|
let url = parts.join('/');
|
|
return url.replace(/(^|[^:])\/\/+/g, '$1/');
|
|
}
|
|
};
|
|
|
|
describe('DynamicRedirectManager', function () {
|
|
it('Prioritizes the query params of the redirect', function () {
|
|
const manager = new DynamicRedirectManager({permanentMaxAge: 100, urlUtils});
|
|
|
|
manager.addRedirect('/test-params', '/result?q=abc', {permanent: true});
|
|
|
|
const req = {
|
|
method: 'GET',
|
|
url: '/test-params/?q=123&lang=js'
|
|
};
|
|
|
|
let headers = null;
|
|
let status = null;
|
|
let location = null;
|
|
const res = {
|
|
set(_headers) {
|
|
headers = _headers;
|
|
},
|
|
redirect(_status, _location) {
|
|
status = _status;
|
|
location = _location;
|
|
}
|
|
};
|
|
|
|
manager.handleRequest(req, res, function next() {
|
|
should.fail(true, false, 'next should not have been called');
|
|
});
|
|
|
|
should.equal(headers['Cache-Control'], 'public, max-age=100');
|
|
should.equal(status, 301);
|
|
should.equal(location, '/result?q=abc&lang=js');
|
|
});
|
|
|
|
it('Allows redirects to be removed', function () {
|
|
const manager = new DynamicRedirectManager({permanentMaxAge: 100, urlUtils});
|
|
|
|
const id = manager.addRedirect('/test-params', '/result?q=abc', {permanent: true});
|
|
manager.removeRedirect(id);
|
|
|
|
const req = {
|
|
method: 'GET',
|
|
url: '/test-params/?q=123&lang=js'
|
|
};
|
|
|
|
let headers = null;
|
|
let status = null;
|
|
let location = null;
|
|
const res = {
|
|
set(_headers) {
|
|
headers = _headers;
|
|
},
|
|
redirect(_status, _location) {
|
|
status = _status;
|
|
location = _location;
|
|
}
|
|
};
|
|
|
|
manager.handleRequest(req, res, function next() {
|
|
should.ok(true, 'next should have been called');
|
|
});
|
|
|
|
should.equal(headers, null);
|
|
should.equal(status, null);
|
|
should.equal(location, null);
|
|
});
|
|
});
|