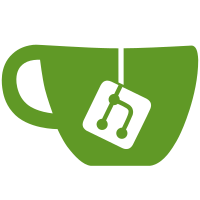
refs: https://github.com/TryGhost/DevOps/issues/11 This is a pretty huge commit, but the relevant points are: * Each importer no longer needs to be passed a set of data, it just gets the data it needs * Each importer specifies its dependencies, so that the order of import can be determined at runtime using a topological sort * The main data generator function can just tell each importer to import the data it has This makes working on the data generator much easier. Some other benefits are: * Batched importing, massively speeding up the whole process * `--tables` to set the exact tables you want to import, and specify the quantity of each
29 lines
905 B
JavaScript
29 lines
905 B
JavaScript
const TableImporter = require('./TableImporter');
|
|
const {faker} = require('@faker-js/faker');
|
|
const {slugify} = require('@tryghost/string');
|
|
const {blogStartDate} = require('../utils/blog-info');
|
|
|
|
class BenefitsImporter extends TableImporter {
|
|
static table = 'benefits';
|
|
static dependencies = [];
|
|
defaultQuantity = 5;
|
|
|
|
constructor(knex, transaction) {
|
|
super(BenefitsImporter.table, knex, transaction);
|
|
}
|
|
|
|
generate() {
|
|
const name = faker.company.catchPhrase();
|
|
const sixMonthsLater = new Date(blogStartDate);
|
|
sixMonthsLater.setMonth(sixMonthsLater.getMonth() + 6);
|
|
return {
|
|
id: faker.database.mongodbObjectId(),
|
|
name: name,
|
|
slug: `${slugify(name)}-${faker.random.numeric(3)}`,
|
|
created_at: faker.date.between(blogStartDate, sixMonthsLater)
|
|
};
|
|
}
|
|
}
|
|
|
|
module.exports = BenefitsImporter;
|