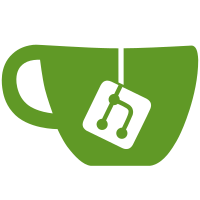
no issue Since `ember-moment@10.0` it's not been necessary to use the `ember-cli-moment-shim` package, with `moment` instead being usable directly via `ember-auto-import`. Getting rid of the shim package is necessary for compatibility with `embroider`, Ember's new build tooling. - dropped `ember-cli-moment-shim` dependency - added `moment-timezone` dependency and updated all imports to reflect the different package - worked around `ember-power-calendar` having `ember-cli-moment-shim` as a sub-dependency - added empty in-repo-addon `ember-power-calendar-moment` to avoid `ember-power-calendar` complaining about a missing package - added `ember-power-calendar-utils` in-repo-addon that is a copy of `ember-power-calendar-moment` but without the build-time renaming of the tree for better compatibility with embroider
38 lines
817 B
JavaScript
38 lines
817 B
JavaScript
import Service from '@ember/service';
|
|
import classic from 'ember-classic-decorator';
|
|
import config from 'ghost-admin/config/environment';
|
|
import moment from 'moment-timezone';
|
|
import {run} from '@ember/runloop';
|
|
|
|
const ONE_SECOND = 1000;
|
|
|
|
// Creates a clock service to run intervals.
|
|
|
|
@classic
|
|
export default class ClockService extends Service {
|
|
second = null;
|
|
minute = null;
|
|
hour = null;
|
|
|
|
init() {
|
|
super.init(...arguments);
|
|
this.tick();
|
|
}
|
|
|
|
tick() {
|
|
let now = moment().utc();
|
|
|
|
this.setProperties({
|
|
second: now.seconds(),
|
|
minute: now.minutes(),
|
|
hour: now.hours()
|
|
});
|
|
|
|
if (config.environment !== 'test') {
|
|
run.later(() => {
|
|
this.tick();
|
|
}, ONE_SECOND);
|
|
}
|
|
}
|
|
}
|