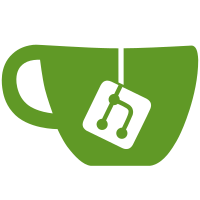
refs https://github.com/TryGhost/Team/issues/584 refs https://github.com/TryGhost/Team/issues/1498 - updated newsletter save routine in `edit-newsletter` modal to open an email confirmation modal if the API indicates one was sent - modal indicates that the previously set or default email will continue to be used until verified - response from API when saving looks like `{newsletters: [{...}], meta: {sent_email_verification: ['sender_name]}}` - added custom newsletter serializer and updated model so that the `meta` property returned in the response when saving posts is exposed - Ember Data only exposes meta on array-response find/query methods - https://github.com/emberjs/data/issues/2905 - added `/settings/members-email-labs/?verifyEmail=xyz` query param handling - opens email verification modal if param is set and instantly clears the query param to avoid problems with sticky params - when the modal opens it makes a `PUT /newsletters/verify-email/` request with the token in the body params, on the API side this works the same as a newsletter update request returning the fully updated newsletter record which is then pushed into the store - removed unused from/reply address code from `<Settings::MembersEmailLabs>` component and controller - setting the values now handled per-newsletter in the edit-newsletter modal - verifying email change is handled in the members-email-labs controller - fixed mirage not outputting pluralized root for "singular" endpoints such as POST/PUT requests to better match our API behaviour
28 lines
797 B
JavaScript
28 lines
797 B
JavaScript
/* eslint-disable camelcase */
|
|
import ApplicationSerializer from './application';
|
|
|
|
export default class MemberSerializer extends ApplicationSerializer {
|
|
// HACK: Ember Data doesn't expose `meta` properties consistently
|
|
// - https://github.com/emberjs/data/issues/2905
|
|
//
|
|
// We need the `meta` data returned when saving so we extract it and dump
|
|
// it onto the model as an attribute then delete it again when serializing.
|
|
normalizeResponse() {
|
|
const json = super.normalizeResponse(...arguments);
|
|
|
|
if (json.meta && json.data.attributes) {
|
|
json.data.attributes._meta = json.meta;
|
|
}
|
|
|
|
return json;
|
|
}
|
|
|
|
serialize() {
|
|
const json = super.serialize(...arguments);
|
|
|
|
delete json._meta;
|
|
|
|
return json;
|
|
}
|
|
}
|