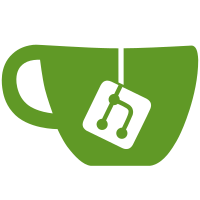
closes: https://github.com/TryGhost/Ghost/issues/15470 - When multiple browser tabs are open, each manipulate a different copy of ember data model, changes to the model in one tab are not reflected in the model of the other tab. - When updating some settings, all current settings were sent to the API. - As a result, when updating two different categories of settings (navigation/code inspection) in different tabs, the second update was overriding the first one. - From a user perspective, this is not a natural behaviour. Only settings visible on-screen when clicking save should be modified. Co-authored-by: Kevin Ansfield <kevin@lookingsideways.co.uk>
29 lines
1.1 KiB
JavaScript
29 lines
1.1 KiB
JavaScript
import ApplicationAdapter from 'ghost-admin/adapters/application';
|
|
import {pluralize} from 'ember-inflector';
|
|
|
|
export default class Setting extends ApplicationAdapter {
|
|
updateRecord(store, type, record) {
|
|
let data = {};
|
|
let serializer = store.serializerFor(type.modelName);
|
|
|
|
// remove the fake id that we added onto the model.
|
|
delete record.id;
|
|
|
|
// use the SettingSerializer to transform the model back into
|
|
// an array of settings objects like the API expects
|
|
serializer.serializeIntoHash(data, type, record);
|
|
|
|
// Do not send empty data to the API
|
|
// This can probably be removed then this is fixed:
|
|
// https://github.com/TryGhost/Ghost/blob/main/ghost/api-framework/lib/validators/input/all.js#L128
|
|
let root = pluralize(type.modelName);
|
|
if (data[root].length === 0) {
|
|
return Promise.resolve();
|
|
}
|
|
|
|
// use the ApplicationAdapter's buildURL method but do not
|
|
// pass in an id.
|
|
return this.ajax(this.buildURL(type.modelName), 'PUT', {data});
|
|
}
|
|
}
|