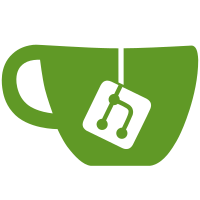
no issue The `settings` service has been a source of confusion when writing with modern Ember patterns because it's use of the deprecated `ProxyMixin` forced all property access/setting to go via `.get()` and `.set()` whereas the rest of the system has mostly (there are a few other uses of ProxyObjects remaining) eliminated the use of the non-native get/set methods. - removed use of `ProxyMixin` in the `settings` service by grabbing the attributes off the setting model after fetching and using `Object.defineProperty()` to add native getters/setters that pass through to the model's getters/setters. Ember's autotracking automatically works across the native getters/setters so we can then use the service as if it was any other native object - updated all code to use `settings.{attrName}` directly for getting/setting instead of `.get()` and `.set()` - removed use of observer in the `customViews` service because it was being set up before the native properties had been added on the settings service meaning autotracking wasn't able to set up properly
40 lines
1.0 KiB
JavaScript
40 lines
1.0 KiB
JavaScript
import Component from '@ember/component';
|
|
import classic from 'ember-classic-decorator';
|
|
import {action, computed} from '@ember/object';
|
|
import {inject as service} from '@ember/service';
|
|
|
|
const VISIBILITIES = [
|
|
{label: 'Public', name: 'public'},
|
|
{label: 'Members only', name: 'members'},
|
|
{label: 'Paid-members only', name: 'paid'}
|
|
];
|
|
|
|
@classic
|
|
export default class GhPsmVisibilityInput extends Component {
|
|
@service settings;
|
|
|
|
// public attrs
|
|
post = null;
|
|
|
|
@computed('post.visibility')
|
|
get selectedVisibility() {
|
|
return this.get('post.visibility') || this.settings.defaultContentVisibility;
|
|
}
|
|
|
|
init() {
|
|
super.init(...arguments);
|
|
this.availableVisibilities = [...VISIBILITIES];
|
|
this.availableVisibilities.push(
|
|
{label: 'Specific tier(s)', name: 'tiers'}
|
|
);
|
|
}
|
|
|
|
@action
|
|
updateVisibility(newVisibility) {
|
|
this.post.set('visibility', newVisibility);
|
|
if (newVisibility !== 'tiers') {
|
|
this.post.set('tiers', []);
|
|
}
|
|
}
|
|
}
|