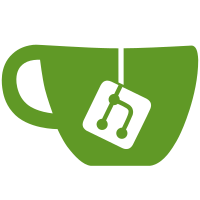
📡 Add debug for the 3 theme activation methods There are 3 different ways that a theme can be activated in Ghost: A. On boot: we load the active theme from the file system, according to the `activeTheme` setting B. On API "activate": when an /activate/ request is triggered for a theme, we validate & change the `activeTheme` setting C. On API "override": if uploading a theme with the same name, we override. Using a dirty hack to make this work. A: setting is done, should load & validate + next request does mounting B: load is done, should validate & change setting + next request does mounting C: load, validate & setting are all done + a hack is needed to ensure the next request does mounting ✨ Validate w/ gscan when theme activating on boot - use the new gscan validation validate.check() method when activating on boot ✨ New concept of active theme - add ActiveTheme class - make it possible to set a theme to be active, and to get the active theme - call the new themes.activate() method in all 3 cases where we activate a theme 🎨 Use new activeTheme to simplify theme code - make use of the new concept where we can, to reduce & simplify code - use new hasPartials() method so we don't have to do file lookups - use path & name getters to reduce use of getContentPath etc - remove requirement on req.app.get('activeTheme') from static-theme middleware (more on this soon) 🚨 Improve theme unit tests (TODO: fix inter-dep) - The theme unit tests are borked! They all pass because they don't test the right things. - This improves them, but they are still dependent on each-other - configHbsForContext tests don't pass if the activateTheme tests aren't run first - I will fix this in a later PR
42 lines
1.2 KiB
JavaScript
42 lines
1.2 KiB
JavaScript
var _ = require('lodash'),
|
|
express = require('express'),
|
|
path = require('path'),
|
|
config = require('../config'),
|
|
themeUtils = require('../themes'),
|
|
utils = require('../utils');
|
|
|
|
function isBlackListedFileType(file) {
|
|
var blackListedFileTypes = ['.hbs', '.md', '.json'],
|
|
ext = path.extname(file);
|
|
return _.includes(blackListedFileTypes, ext);
|
|
}
|
|
|
|
function isWhiteListedFile(file) {
|
|
var whiteListedFiles = ['manifest.json'],
|
|
base = path.basename(file);
|
|
return _.includes(whiteListedFiles, base);
|
|
}
|
|
|
|
function forwardToExpressStatic(req, res, next) {
|
|
if (!themeUtils.getActive()) {
|
|
next();
|
|
} else {
|
|
var configMaxAge = config.get('caching:theme:maxAge');
|
|
|
|
express.static(themeUtils.getActive().path,
|
|
{maxAge: (configMaxAge || configMaxAge === 0) ? configMaxAge : utils.ONE_YEAR_MS}
|
|
)(req, res, next);
|
|
}
|
|
}
|
|
|
|
function staticTheme() {
|
|
return function blackListStatic(req, res, next) {
|
|
if (!isWhiteListedFile(req.path) && isBlackListedFileType(req.path)) {
|
|
return next();
|
|
}
|
|
return forwardToExpressStatic(req, res, next);
|
|
};
|
|
}
|
|
|
|
module.exports = staticTheme;
|