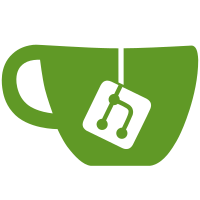
* 🔥 kill apiUrl helper, use urlFor helper instead More consistency of creating urls. Creates an easier ability to add config changes. Attention: urlFor function is getting a little nesty, BUT that is for now wanted to make easier and centralised changes to the configs. The url util need's refactoring anyway. * 🔥 urlSSL Remove all urlSSL usages. Add TODO's for the next commit to re-add logic for deleted logic. e.g. - cors helper generated an array of url's to allow requests from the defined config url's -> will be replaced by the admin url if available - theme handler prefered the urlSSL in case it was defined -> will be replaced by using the urlFor helper to get the blog url (based on the request secure flag) The changes in this commit doesn't have to be right, but it helped going step by step. The next commit is the more interesting one. * 🔥 ✨ remove forceAdminSSL, add new admin url and adapt logic I wanted to remove the forceAdminSSL as separate commit, but was hard to realise. That's why both changes are in one commit: 1. remove forceAdminSSL 2. add admin.url option - fix TODO's from last commits - rewrite the ssl middleware! - create some private helper functions in the url helper to realise the changes - rename some wordings and functions e.g. base === blog (we have so much different wordings) - i would like to do more, but this would end in a non readable PR - this commit contains the most important changes to offer admin.url option * 🤖 adapt tests IMPORTANT - all changes in the routing tests were needed, because each routing test did not start the ghost server - they just required the ghost application, which resulted in a random server port - having a random server port results in a redirect, caused by the ssl/redirect middleware * 😎 rename check-ssl middleware * 🎨 fix theme-handler because of master rebase
89 lines
2.1 KiB
JavaScript
89 lines
2.1 KiB
JavaScript
var cors = require('cors'),
|
|
_ = require('lodash'),
|
|
url = require('url'),
|
|
os = require('os'),
|
|
utils = require('../../utils'),
|
|
whitelist = [],
|
|
ENABLE_CORS = {origin: true, maxAge: 86400},
|
|
DISABLE_CORS = {origin: false};
|
|
|
|
/**
|
|
* Gather a list of local ipv4 addresses
|
|
* @return {Array<String>}
|
|
*/
|
|
function getIPs() {
|
|
var ifaces = os.networkInterfaces(),
|
|
ips = [
|
|
'localhost'
|
|
];
|
|
|
|
Object.keys(ifaces).forEach(function (ifname) {
|
|
ifaces[ifname].forEach(function (iface) {
|
|
// only support IPv4
|
|
if (iface.family !== 'IPv4') {
|
|
return;
|
|
}
|
|
|
|
ips.push(iface.address);
|
|
});
|
|
});
|
|
|
|
return ips;
|
|
}
|
|
|
|
function getUrls() {
|
|
var blogHost = url.parse(utils.url.urlFor('home', true)).hostname,
|
|
adminHost = url.parse(utils.url.urlFor('admin', true)).hostname,
|
|
urls = [];
|
|
|
|
urls.push(blogHost);
|
|
|
|
if (adminHost !== blogHost) {
|
|
urls.push(adminHost);
|
|
}
|
|
|
|
return urls;
|
|
}
|
|
|
|
function getWhitelist() {
|
|
// This needs doing just one time after init
|
|
if (_.isEmpty(whitelist)) {
|
|
// origins that always match: localhost, local IPs, etc.
|
|
whitelist = whitelist.concat(getIPs());
|
|
// Trusted urls from config.js
|
|
whitelist = whitelist.concat(getUrls());
|
|
}
|
|
|
|
return whitelist;
|
|
}
|
|
|
|
/**
|
|
* Checks the origin and enables/disables CORS headers in the response.
|
|
* @param {Object} req express request object.
|
|
* @param {Function} cb callback that configures CORS.
|
|
* @return {null}
|
|
*/
|
|
function handleCORS(req, cb) {
|
|
var origin = req.get('origin'),
|
|
trustedDomains = req.client && req.client.trustedDomains;
|
|
|
|
// Request must have an Origin header
|
|
if (!origin) {
|
|
return cb(null, DISABLE_CORS);
|
|
}
|
|
|
|
// Origin matches a client_trusted_domain
|
|
if (_.some(trustedDomains, {trusted_domain: origin})) {
|
|
return cb(null, ENABLE_CORS);
|
|
}
|
|
|
|
// Origin matches whitelist
|
|
if (getWhitelist().indexOf(url.parse(origin).hostname) > -1) {
|
|
return cb(null, ENABLE_CORS);
|
|
}
|
|
|
|
return cb(null, DISABLE_CORS);
|
|
}
|
|
|
|
module.exports = cors(handleCORS);
|