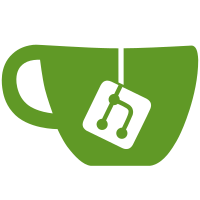
closes #367 closes #368 - Adds Tag model with a many-to-many relationship with Post - Adds Tag API to retrieve all previously used Tags (needed for suggestions) - Allows setting and retrieval of Tags for a post through the Post's existing API endpoints. - Hooks up the editor's tag suggestion box to the Ghost install's previously used tags - Tidies the client code for adding tags, and encapsulates the functionality into a Backbone view
64 lines
1.8 KiB
JavaScript
64 lines
1.8 KiB
JavaScript
/*global window, document, Ghost, $, _, Backbone */
|
|
(function () {
|
|
"use strict";
|
|
|
|
Ghost.Models.Post = Backbone.Model.extend({
|
|
|
|
defaults: {
|
|
status: 'draft'
|
|
},
|
|
|
|
blacklist: ['published', 'draft'],
|
|
|
|
parse: function (resp) {
|
|
if (resp.status) {
|
|
resp.published = !!(resp.status === "published");
|
|
resp.draft = !!(resp.status === "draft");
|
|
}
|
|
if (resp.tags) {
|
|
// TODO: parse tags into it's own collection on the model (this.tags)
|
|
return resp;
|
|
}
|
|
return resp;
|
|
},
|
|
|
|
validate: function (attrs) {
|
|
if (_.isEmpty(attrs.title)) {
|
|
return 'You must specify a title for the post.';
|
|
}
|
|
},
|
|
|
|
addTag: function (tagToAdd) {
|
|
var tags = this.get('tags') || [];
|
|
tags.push(tagToAdd);
|
|
this.set('tags', tags);
|
|
},
|
|
|
|
removeTag: function (tagToRemove) {
|
|
var tags = this.get('tags') || [];
|
|
tags = _.reject(tags, function (tag) {
|
|
return tag.id === tagToRemove.id || tag.name === tagToRemove.name;
|
|
});
|
|
this.set('tags', tags);
|
|
}
|
|
});
|
|
|
|
Ghost.Collections.Posts = Backbone.Collection.extend({
|
|
url: Ghost.settings.apiRoot + '/posts',
|
|
model: Ghost.Models.Post,
|
|
parse: function (resp) {
|
|
if (_.isArray(resp.posts)) {
|
|
this.limit = resp.limit;
|
|
this.currentPage = resp.page;
|
|
this.totalPages = resp.pages;
|
|
this.totalPosts = resp.total;
|
|
this.nextPage = resp.next;
|
|
this.prevPage = resp.prev;
|
|
return resp.posts;
|
|
}
|
|
return resp;
|
|
}
|
|
});
|
|
|
|
}());
|