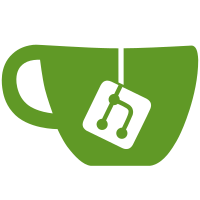
refs https://github.com/TryGhost/Product/issues/3504 - When you are logged in as an admin, but not as a member, no buttons showed (discovered in new e2e tests) - Added E2E tests for admin actions
168 lines
5.4 KiB
TypeScript
168 lines
5.4 KiB
TypeScript
import {MOCKED_SITE_URL, MockedApi, initialize, mockAdminAuthFrame} from '../utils/e2e';
|
|
import {expect, test} from '@playwright/test';
|
|
|
|
const admin = MOCKED_SITE_URL + '/ghost/';
|
|
|
|
test.describe('Auth Frame', async () => {
|
|
test('renders the auth frame', async ({page}) => {
|
|
const mockedApi = new MockedApi({});
|
|
await initialize({
|
|
mockedApi,
|
|
page,
|
|
publication: 'Publisher Weekly',
|
|
admin
|
|
});
|
|
|
|
const iframeElement = await page.locator('iframe[data-frame="admin-auth"]');
|
|
await expect(iframeElement).toHaveCount(1);
|
|
});
|
|
|
|
test('has no admin options', async ({page}) => {
|
|
const mockedApi = new MockedApi({});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 1</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 2</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 3</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 4</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 5</p>'
|
|
});
|
|
|
|
const {frame} = await initialize({
|
|
mockedApi,
|
|
page,
|
|
publication: 'Publisher Weekly',
|
|
admin
|
|
});
|
|
|
|
const iframeElement = await page.locator('iframe[data-frame="admin-auth"]');
|
|
await expect(iframeElement).toHaveCount(1);
|
|
|
|
const comments = await frame.getByTestId('comment-component');
|
|
await expect(comments).toHaveCount(5);
|
|
|
|
const moreButtons = await frame.getByTestId('more-button');
|
|
await expect(moreButtons).toHaveCount(0);
|
|
});
|
|
|
|
test('has admin options when signed in to Ghost admin', async ({page}) => {
|
|
const mockedApi = new MockedApi({});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 1</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 2</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 3</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 4</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 5</p>'
|
|
});
|
|
|
|
await mockAdminAuthFrame({
|
|
admin,
|
|
page
|
|
});
|
|
|
|
const {frame} = await initialize({
|
|
mockedApi,
|
|
page,
|
|
publication: 'Publisher Weekly',
|
|
admin
|
|
});
|
|
|
|
const iframeElement = await page.locator('iframe[data-frame="admin-auth"]');
|
|
await expect(iframeElement).toHaveCount(1);
|
|
|
|
// Check if more actions button is visible on each comment
|
|
const comments = await frame.getByTestId('comment-component');
|
|
await expect(comments).toHaveCount(5);
|
|
|
|
const moreButtons = await frame.getByTestId('more-button');
|
|
await expect(moreButtons).toHaveCount(5);
|
|
|
|
// Click the 2nd button
|
|
await moreButtons.nth(1).click();
|
|
await moreButtons.nth(1).getByText('Hide comment').click();
|
|
|
|
// Check comment2 is replaced with a hidden message
|
|
const secondComment = comments.nth(1);
|
|
await expect(secondComment).toHaveText('This comment has been hidden.');
|
|
await expect(secondComment).not.toContainText('This is comment 4');
|
|
|
|
// Check can show it again
|
|
await moreButtons.nth(1).click();
|
|
await moreButtons.nth(1).getByText('Show comment').click();
|
|
await expect(secondComment).toContainText('This is comment 4');
|
|
});
|
|
|
|
test('has admin options when signed in to Ghost admin and as a member', async ({page}) => {
|
|
const mockedApi = new MockedApi({});
|
|
mockedApi.setMember({});
|
|
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 1</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 2</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 3</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 4</p>'
|
|
});
|
|
mockedApi.addComment({
|
|
html: '<p>This is comment 5</p>'
|
|
});
|
|
|
|
await mockAdminAuthFrame({
|
|
admin,
|
|
page
|
|
});
|
|
|
|
const {frame} = await initialize({
|
|
mockedApi,
|
|
page,
|
|
publication: 'Publisher Weekly',
|
|
admin
|
|
});
|
|
|
|
const iframeElement = await page.locator('iframe[data-frame="admin-auth"]');
|
|
await expect(iframeElement).toHaveCount(1);
|
|
|
|
// Check if more actions button is visible on each comment
|
|
const comments = await frame.getByTestId('comment-component');
|
|
await expect(comments).toHaveCount(5);
|
|
|
|
const moreButtons = await frame.getByTestId('more-button');
|
|
await expect(moreButtons).toHaveCount(5);
|
|
|
|
// Click the 2nd button
|
|
await moreButtons.nth(1).click();
|
|
await moreButtons.nth(1).getByText('Hide comment').click();
|
|
|
|
// Check comment2 is replaced with a hidden message
|
|
const secondComment = comments.nth(1);
|
|
await expect(secondComment).toHaveText('This comment has been hidden.');
|
|
await expect(secondComment).not.toContainText('This is comment 4');
|
|
|
|
// Check can show it again
|
|
await moreButtons.nth(1).click();
|
|
await moreButtons.nth(1).getByText('Show comment').click();
|
|
await expect(secondComment).toContainText('This is comment 4');
|
|
});
|
|
});
|
|
|