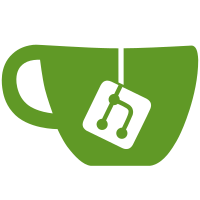
refs https://github.com/TryGhost/Team/issues/1277 - `data-cache` service has a `.set(key, data, lifetime)` method that will store the data under the key and sets a timeout that will remove the data when the lifetime expires - data can be retrieved with `.get(key)` - allows for components to cache data for use when re-rendering without having to worry about keeping track of their state and it's expiration manually somewhere else - moved caching concern out of the `members-activity` service and into the latest-member-activity dashboard component which is the one that cares about it's data and cache lifetime - frees the `members-activity` service up to be more generic as it's no longer tied to the dashboard component's concerns - component switched to using a task rather than a promise so it is automatically cancelled if it's destroyed before data fetching is complete
38 lines
784 B
JavaScript
38 lines
784 B
JavaScript
import Service from '@ember/service';
|
|
|
|
const ONE_MINUTE = 1 * 60 * 1000;
|
|
|
|
export default class DataCacheService extends Service {
|
|
cache = {};
|
|
timeouts = {};
|
|
|
|
get(key) {
|
|
return this.cache[key];
|
|
}
|
|
|
|
set(key, data, lifetime = ONE_MINUTE) {
|
|
this.cache[key] = data;
|
|
|
|
this.timeouts[key] = window.setTimeout(() => {
|
|
delete this.cache[key];
|
|
delete this.timeouts[key];
|
|
}, lifetime);
|
|
|
|
return this.cache[key];
|
|
}
|
|
|
|
clear() {
|
|
this._clearAllTimeouts();
|
|
this.cache = {};
|
|
this.timeouts = {};
|
|
}
|
|
|
|
willDestroy() {
|
|
this._clearAllTimeouts();
|
|
}
|
|
|
|
_clearAllTimeouts() {
|
|
Object.keys(this.timeouts).forEach(key => window.clearTimeout(this.timeouts[key]));
|
|
}
|
|
}
|