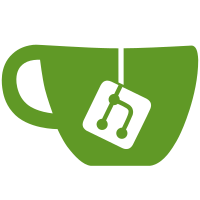
- 🛠 add bunyan and prettyjson, remove morgan - ✨ add logging module - GhostLogger class that handles setup of bunyan - PrettyStream for stdout - ✨ config for logging - @TODO: testing level fatal? - ✨ log each request via GhostLogger (express middleware) - @TODO: add errors to output - 🔥 remove errors.updateActiveTheme - we can read the value from config - 🔥 remove 15 helper functions in core/server/errors/index.js - all these functions get replaced by modules: 1. logging 2. error middleware handling for html/json 3. error creation (which will be part of PR #7477) - ✨ add express error handler for html/json - one true error handler for express responses - contains still some TODO's, but they are not high priority for first implementation/integration - this middleware only takes responsibility of either rendering html responses or return json error responses - 🎨 use new express error handler in middleware/index - 404 and 500 handling - 🎨 return error instead of error message in permissions/index.js - the rule for error handling should be: if you call a unit, this unit should return a custom Ghost error - 🎨 wrap serve static module - rule: if you call a module/unit, you should always wrap this error - it's always the same rule - so the caller never has to worry about what comes back - it's always a clear error instance - in this case: we return our notfounderror if serve static does not find the resource - this avoid having checks everywhere - 🎨 replace usages of errors/index.js functions and adapt tests - use logging.error, logging.warn - make tests green - remove some usages of logging and throwing api errors -> because when a request is involved, logging happens automatically - 🐛 return errorDetails to Ghost-Admin - errorDetails is used for Theme error handling - 🎨 use 500er error for theme is missing error in theme-handler - 🎨 extend file rotation to 1w
111 lines
3.4 KiB
JavaScript
111 lines
3.4 KiB
JavaScript
var _ = require('lodash'),
|
|
path = require('path'),
|
|
hbs = require('express-hbs'),
|
|
config = require('../config'),
|
|
i18n = require('../i18n'),
|
|
_private = {};
|
|
|
|
_private.parseStack = function (stack) {
|
|
if (!_.isString(stack)) {
|
|
return stack;
|
|
}
|
|
|
|
// TODO: split out line numbers
|
|
var stackRegex = /\s*at\s*(\w+)?\s*\(([^\)]+)\)\s*/i;
|
|
|
|
return (
|
|
stack
|
|
.split(/[\r\n]+/)
|
|
.slice(1)
|
|
.map(function (line) {
|
|
var parts = line.match(stackRegex);
|
|
if (!parts) {
|
|
return null;
|
|
}
|
|
|
|
return {
|
|
function: parts[1],
|
|
at: parts[2]
|
|
};
|
|
})
|
|
.filter(function (line) {
|
|
return !!line;
|
|
})
|
|
);
|
|
};
|
|
|
|
_private.handleHTMLResponse = function handleHTMLResponse(err, req, res) {
|
|
return function handleHTMLResponse() {
|
|
var availableTheme = config.get('paths').availableThemes[req.app.get('activeTheme')] || {},
|
|
defaultTemplate = availableTheme['error.hbs'] ||
|
|
path.resolve(config.get('paths').adminViews, 'user-error.hbs') ||
|
|
'error';
|
|
|
|
res.render(defaultTemplate, {
|
|
message: err.message,
|
|
code: err.statusCode,
|
|
stack: _private.parseStack(err.stack)
|
|
}, function renderResponse(err, html) {
|
|
if (!err) {
|
|
return res.send(html);
|
|
}
|
|
|
|
// And then try to explain things to the user...
|
|
// Cheat and output the error using handlebars escapeExpression
|
|
return res.status(500).send(
|
|
'<h1>' + i18n.t('errors.errors.oopsErrorTemplateHasError') + '</h1>' +
|
|
'<p>' + i18n.t('errors.errors.encounteredError') + '</p>' +
|
|
'<pre>' + hbs.handlebars.Utils.escapeExpression(err.message || err) + '</pre>' +
|
|
'<br ><p>' + i18n.t('errors.errors.whilstTryingToRender') + '</p>' +
|
|
err.statusCode + ' ' + '<pre>' + hbs.handlebars.Utils.escapeExpression(err.message || err) + '</pre>'
|
|
);
|
|
});
|
|
};
|
|
};
|
|
|
|
/**
|
|
* @TODO: jsonapi errors format
|
|
*/
|
|
_private.handleJSONResponse = function handleJSONResponse(err, req, res) {
|
|
return function handleJSONResponse() {
|
|
res.json({
|
|
errors: [{
|
|
message: err.message,
|
|
errorType: err.errorType,
|
|
errorDetails: err.errorDetails
|
|
}]
|
|
});
|
|
};
|
|
};
|
|
|
|
/**
|
|
* @TODO: test uncaught exception (wrap into custom error!)
|
|
* @TODO: support multiple errors
|
|
* @TODO: decouple req.err
|
|
*/
|
|
module.exports = function errorHandler(err, req, res, next) {
|
|
if (_.isArray(err)) {
|
|
err = err[0];
|
|
}
|
|
|
|
req.err = err;
|
|
res.statusCode = err.statusCode;
|
|
|
|
// never cache errors
|
|
res.set({
|
|
'Cache-Control': 'no-cache, private, no-store, must-revalidate, max-stale=0, post-check=0, pre-check=0'
|
|
});
|
|
|
|
// @TODO: does this resolves all use cases?
|
|
if (!req.headers.accept && !req.headers.hasOwnProperty('content-type')) {
|
|
req.headers.accept = 'text/html';
|
|
}
|
|
|
|
// jscs:disable
|
|
res.format({
|
|
json: _private.handleJSONResponse(err, req, res, next),
|
|
html: _private.handleHTMLResponse(err, req, res, next),
|
|
'default': _private.handleHTMLResponse(err, req, res, next)
|
|
});
|
|
};
|