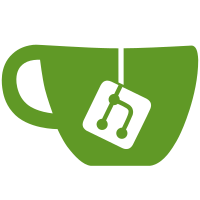
closes https://github.com/TryGhost/Arch/issues/91
- We have on cascade delete (a9f9f6121a/ghost/core/core/server/data/schema/schema.js (L1068)
) on `post_id` column which handles post deletion logic automatically on DB level.
- The commented out handlers in the long term should be hooked up with public CollectionService methods on the client side.
48 lines
1.5 KiB
TypeScript
48 lines
1.5 KiB
TypeScript
import assert from 'assert/strict';
|
|
import {
|
|
PostDeletedEvent,
|
|
PostsBulkDestroyedEvent,
|
|
PostsBulkUnpublishedEvent,
|
|
PostsBulkFeaturedEvent,
|
|
PostsBulkUnfeaturedEvent,
|
|
PostsBulkAddTagsEvent
|
|
} from '../src/index';
|
|
|
|
describe('Post Events', function () {
|
|
it('Can instantiate PostDeletedEvent', function () {
|
|
const event = PostDeletedEvent.create({id: 'post-id-1', data: {}});
|
|
assert.ok(event);
|
|
assert.equal(event.id, 'post-id-1');
|
|
});
|
|
|
|
it('Can instantiate BulkDestroyEvent', function () {
|
|
const event = PostsBulkDestroyedEvent.create(['1', '2', '3']);
|
|
assert.ok(event);
|
|
assert.equal(event.data.length, 3);
|
|
});
|
|
|
|
it('Can instantiate PostsBulkUnpublishedEvent', function () {
|
|
const event = PostsBulkUnpublishedEvent.create(['1', '2', '3']);
|
|
assert.ok(event);
|
|
assert.equal(event.data.length, 3);
|
|
});
|
|
|
|
it('Can instantiate PostsBulkFeaturedEvent', function () {
|
|
const event = PostsBulkFeaturedEvent.create(['1', '2', '3']);
|
|
assert.ok(event);
|
|
assert.equal(event.data.length, 3);
|
|
});
|
|
|
|
it('Can instantiate PostsBulkUnfeaturedEvent', function () {
|
|
const event = PostsBulkUnfeaturedEvent.create(['1', '2', '3']);
|
|
assert.ok(event);
|
|
assert.equal(event.data.length, 3);
|
|
});
|
|
|
|
it('Can instantiate PostsBulkAddTagsEvent', function () {
|
|
const event = PostsBulkAddTagsEvent.create(['1', '2', '3']);
|
|
assert.ok(event);
|
|
assert.equal(event.data.length, 3);
|
|
});
|
|
});
|