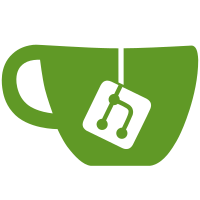
refs b6728ecb0f
- The "no-shadow" eslint rune was introduced into ghost's eslint plugin (referenced commmit), which resulted in flood of warning in console output when linting the project codebase.
- This cleanup is aiming to make any new linting issues more visible. Follow up commits will contain similar cleanups in other parts of the codebase
42 lines
1.1 KiB
JavaScript
42 lines
1.1 KiB
JavaScript
const _ = require('lodash');
|
|
const config = require('../../core/shared/config');
|
|
const configUtils = {};
|
|
|
|
configUtils.config = config;
|
|
configUtils.defaultConfig = _.cloneDeep(config.get());
|
|
|
|
/**
|
|
* configUtils.set({});
|
|
* configUtils.set('key', 'value');
|
|
*/
|
|
configUtils.set = function () {
|
|
const key = arguments[0];
|
|
const value = arguments[1];
|
|
|
|
if (_.isObject(key)) {
|
|
_.each(key, function (settingValue, settingKey) {
|
|
config.set(settingKey, settingValue);
|
|
});
|
|
} else {
|
|
config.set(key, value);
|
|
}
|
|
};
|
|
|
|
/**
|
|
* important: do not delete cloneDeep for value
|
|
* nconf keeps this as a reference and then it can happen that the defaultConfig get's overridden by new values
|
|
*/
|
|
configUtils.restore = function () {
|
|
/**
|
|
* we have to reset the whole config object
|
|
* config keys, which get set via a test and do not exist in the config files, won't get reseted
|
|
*/
|
|
config.reset();
|
|
|
|
_.each(configUtils.defaultConfig, function (value, key) {
|
|
config.set(key, _.cloneDeep(value));
|
|
});
|
|
};
|
|
|
|
module.exports = configUtils;
|