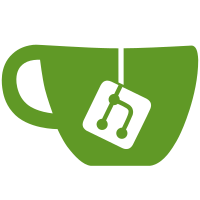
refs TryGhost/Product#4175 - Added error handling to Sentry's beforeSend function in both Admin and Core, so if there is any error in beforeSend, we will still send the unmodified event to Sentry - This is in response to an incident yesterday wherein the beforeSend function threw an error due to an unexpected missing value in the exception. The event sent to Sentry was the error in the beforeSend function, and the original error never reached Sentry. - If the original event had reached Sentry, even if unmodified by the logic in beforeSend, we could have been alerted to the issue sooner and more easily identified all affected sites. - Also added defensive logic to protect for certain values in the exception passed to beforeSend not existing and added unit tests for the beforeSend function in admin and core
43 lines
1.7 KiB
JavaScript
43 lines
1.7 KiB
JavaScript
import {
|
|
isAjaxError
|
|
} from 'ember-ajax/errors';
|
|
|
|
export function beforeSend(event, hint) {
|
|
try {
|
|
const exception = hint.originalException;
|
|
event.tags = event.tags || {};
|
|
event.tags.shown_to_user = event.tags.shown_to_user || false;
|
|
event.tags.grammarly = !!document.querySelector('[data-gr-ext-installed]');
|
|
|
|
// Do not report "handled" errors to Sentry
|
|
if (event.tags.shown_to_user === true) {
|
|
return null;
|
|
}
|
|
|
|
// if the error value includes a model id then overwrite it to improve grouping
|
|
if (event.exception && event.exception.values && event.exception.values.length > 0) {
|
|
const pattern = /<(post|page):[a-f0-9]+>/;
|
|
const replacement = '<$1:ID>';
|
|
event.exception.values[0].value = event.exception.values[0].value.replace(pattern, replacement);
|
|
}
|
|
|
|
// ajax errors — improve logging and add context for debugging
|
|
if (isAjaxError(exception) && exception.payload && exception.payload.errors && exception.payload.errors.length > 0) {
|
|
const error = exception.payload.errors[0];
|
|
event.exception.values[0].type = `${error.type}: ${error.context}`;
|
|
event.exception.values[0].value = error.message;
|
|
event.exception.values[0].context = error.context;
|
|
} else {
|
|
delete event.contexts.ajax;
|
|
delete event.tags.ajax_status;
|
|
delete event.tags.ajax_method;
|
|
delete event.tags.ajax_url;
|
|
}
|
|
|
|
return event;
|
|
} catch (error) {
|
|
// If any errors occur in beforeSend, send the original event to Sentry
|
|
// Better to have some information than no information
|
|
return event;
|
|
}
|
|
} |