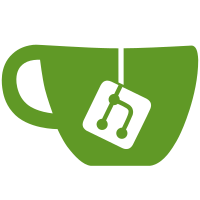
closes: https://github.com/TryGhost/Team/issues/1203 refs: https://github.com/TryGhost/Ghost/issues/9942 - Ensures that the webhook secret is validated and saved in Ghost admin - Then makes use of this value by optionally adding an X-Ghost-Signature header that effectively signs the webhooks - This allows for verifying the source of a webhook coming from Ghost is truly Ghost. - Uses the same pattern as GitHub uses: https://docs.github.com/en/developers/webhooks-and-events/webhooks/securing-your-webhooks Co-authored-by: Hannah Wolfe <github.erisds@gmail.com>
52 lines
1.7 KiB
JavaScript
52 lines
1.7 KiB
JavaScript
import BaseValidator from './base';
|
|
import validator from 'validator';
|
|
import {isBlank} from '@ember/utils';
|
|
|
|
export default BaseValidator.create({
|
|
properties: ['name', 'event', 'targetUrl', 'secret'],
|
|
|
|
name(model) {
|
|
if (isBlank(model.name)) {
|
|
model.errors.add('name', 'Please enter a name');
|
|
model.hasValidated.pushObject('name');
|
|
this.invalidate();
|
|
} else if (!validator.isLength(model.name, 0, 191)) {
|
|
model.errors.add('name', 'Name is too long, max 191 chars');
|
|
model.hasValidated.pushObject('name');
|
|
this.invalidate();
|
|
}
|
|
},
|
|
|
|
event(model) {
|
|
if (isBlank(model.event)) {
|
|
model.errors.add('event', 'Please select an event');
|
|
model.hasValidated.pushObject('event');
|
|
this.invalidate();
|
|
}
|
|
},
|
|
|
|
targetUrl(model) {
|
|
if (isBlank(model.targetUrl)) {
|
|
model.errors.add('targetUrl', 'Please enter a target URL');
|
|
} else if (!validator.isURL(model.targetUrl || '', {require_protocol: false})) {
|
|
model.errors.add('targetUrl', 'Please enter a valid URL');
|
|
} else if (!validator.isLength(model.targetUrl, 0, 2000)) {
|
|
model.errors.add('targetUrl', 'Target URL is too long, max 2000 chars');
|
|
}
|
|
|
|
model.hasValidated.pushObject('targetUrl');
|
|
|
|
if (model.errors.has('targetUrl')) {
|
|
this.invalidate();
|
|
}
|
|
},
|
|
|
|
secret(model) {
|
|
if (!isBlank(model.secret) && !validator.isLength(model.secret, 0, 191)) {
|
|
model.errors.add('secret', 'Secret is too long, max 191 chars');
|
|
model.hasValidated.pushObject('secret');
|
|
this.invalidate();
|
|
}
|
|
}
|
|
});
|