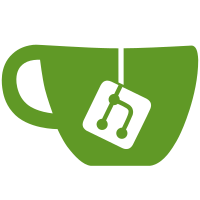
- Created Role model - Created Permission model - Linked Users->Roles with a belongsToMany relationship - Linked Permissions to Users and Roles with a belongsToMany relationship - Created permissions helper with functions for initializing and checking permissions (canThis) - Unit tests for lots of things
31 lines
648 B
JavaScript
31 lines
648 B
JavaScript
(function () {
|
|
"use strict";
|
|
|
|
var GhostBookshelf = require('./base'),
|
|
User = require('./user').User,
|
|
Role = require('./role').Role,
|
|
Permission,
|
|
Permissions;
|
|
|
|
Permission = GhostBookshelf.Model.extend({
|
|
tableName: 'permissions',
|
|
|
|
roles: function () {
|
|
return this.belongsToMany(Role);
|
|
},
|
|
|
|
users: function () {
|
|
return this.belongsToMany(User);
|
|
}
|
|
});
|
|
|
|
Permissions = GhostBookshelf.Collection.extend({
|
|
model: Permission
|
|
});
|
|
|
|
module.exports = {
|
|
Permission: Permission,
|
|
Permissions: Permissions
|
|
};
|
|
|
|
}()); |