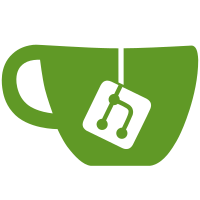
refs https://github.com/TryGhost/Team/issues/2077 - The "productRepository" methods have been deprecated in favor of "tiers" and "Tiers API". - The changes migrated usages of "productRepository.getDefaultProduct" to Tiers API's "readDefaultTier"
66 lines
1.5 KiB
JavaScript
66 lines
1.5 KiB
JavaScript
const nql = require('@tryghost/nql');
|
|
|
|
/**
|
|
* @typedef {import('./Tier')} Tier
|
|
*/
|
|
|
|
class InMemoryTierRepository {
|
|
/** @type {Tier[]} */
|
|
#store = [];
|
|
/** @type {Object.<string, true>} */
|
|
#ids = {};
|
|
|
|
/**
|
|
* @param {Tier} tier
|
|
* @returns {any}
|
|
*/
|
|
toPrimitive(tier) {
|
|
return {
|
|
...tier,
|
|
active: (tier.status === 'active'),
|
|
type: tier.type,
|
|
id: tier.id.toHexString()
|
|
};
|
|
}
|
|
|
|
/**
|
|
* @param {Tier} linkClick
|
|
* @returns {Promise<void>}
|
|
*/
|
|
async save(tier) {
|
|
if (this.#ids[tier.id.toHexString()]) {
|
|
const existing = this.#store.findIndex((item) => {
|
|
return item.id.equals(tier.id);
|
|
});
|
|
this.#store.splice(existing, 1, tier);
|
|
} else {
|
|
this.#store.push(tier);
|
|
this.#ids[tier.id.toHexString()] = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param {import('bson-objectid').default} id
|
|
* @returns {Promise<Tier>}
|
|
*/
|
|
async getById(id) {
|
|
return this.#store.find((item) => {
|
|
return item.id.equals(id);
|
|
});
|
|
}
|
|
|
|
/**
|
|
* @param {object} [options]
|
|
* @param {string} [options.filter]
|
|
* @returns {Promise<Tier[]>}
|
|
*/
|
|
async getAll(options = {}) {
|
|
const filter = nql(options.filter, {});
|
|
return this.#store.slice().filter((item) => {
|
|
return filter.queryJSON(this.toPrimitive(item));
|
|
});
|
|
}
|
|
}
|
|
|
|
module.exports = InMemoryTierRepository;
|