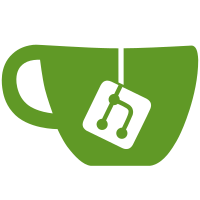
closes https://linear.app/tryghost/issue/PLG-15 - removed `internalLinking` GA labs flag - renamed search providers to `flex` and `basic` - keeps old search provider around as it can handle non-English languages unlike the faster flex provider - updated `search` service to switch from `flex` to `basic` when the site's locale is not english - bumped Koenig packages to switch from a feature flag for toggling internal linking features to the presence of the `searchLinks` function in card config - updated tests to correctly switch between flex and basic providers in respective suites
61 lines
1.6 KiB
JavaScript
61 lines
1.6 KiB
JavaScript
import Service from '@ember/service';
|
|
import {action} from '@ember/object';
|
|
import {isBlank} from '@ember/utils';
|
|
import {inject as service} from '@ember/service';
|
|
import {task, timeout} from 'ember-concurrency';
|
|
|
|
export default class SearchService extends Service {
|
|
@service ajax;
|
|
@service feature;
|
|
@service notifications;
|
|
@service searchProviderBasic;
|
|
@service searchProviderFlex;
|
|
@service settings;
|
|
@service store;
|
|
|
|
isContentStale = true;
|
|
|
|
get provider() {
|
|
const isEnglish = this.settings.locale?.toLowerCase().startsWith('en') ?? true;
|
|
return isEnglish ? this.searchProviderFlex : this.searchProviderBasic;
|
|
}
|
|
|
|
@action
|
|
expireContent() {
|
|
this.isContentStale = true;
|
|
}
|
|
|
|
@task({restartable: true})
|
|
*searchTask(term) {
|
|
if (isBlank(term)) {
|
|
return [];
|
|
}
|
|
|
|
// start loading immediately in the background
|
|
this.refreshContentTask.perform();
|
|
|
|
// debounce searches to 200ms to avoid thrashing CPU
|
|
yield timeout(200);
|
|
|
|
// wait for any on-going refresh to finish
|
|
if (this.refreshContentTask.isRunning) {
|
|
yield this.refreshContentTask.lastRunning;
|
|
}
|
|
|
|
return yield this.provider.searchTask.perform(term);
|
|
}
|
|
|
|
@task({drop: true})
|
|
*refreshContentTask({forceRefresh = false} = {}) {
|
|
if (!forceRefresh && !this.isContentStale) {
|
|
return true;
|
|
}
|
|
|
|
this.isContentStale = true;
|
|
|
|
yield this.provider.refreshContentTask.perform();
|
|
|
|
this.isContentStale = false;
|
|
}
|
|
}
|