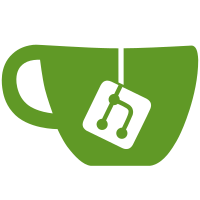
no issue The `settings` service has been a source of confusion when writing with modern Ember patterns because it's use of the deprecated `ProxyMixin` forced all property access/setting to go via `.get()` and `.set()` whereas the rest of the system has mostly (there are a few other uses of ProxyObjects remaining) eliminated the use of the non-native get/set methods. - removed use of `ProxyMixin` in the `settings` service by grabbing the attributes off the setting model after fetching and using `Object.defineProperty()` to add native getters/setters that pass through to the model's getters/setters. Ember's autotracking automatically works across the native getters/setters so we can then use the service as if it was any other native object - updated all code to use `settings.{attrName}` directly for getting/setting instead of `.get()` and `.set()` - removed use of observer in the `customViews` service because it was being set up before the native properties had been added on the settings service meaning autotracking wasn't able to set up properly
64 lines
2.1 KiB
JavaScript
64 lines
2.1 KiB
JavaScript
import Component from '@glimmer/component';
|
|
import {action} from '@ember/object';
|
|
|
|
export default class GhTwitterUrlInput extends Component {
|
|
get value() {
|
|
const {model, modelProperty, scratchValue} = this.args;
|
|
return scratchValue || model[modelProperty];
|
|
}
|
|
|
|
@action
|
|
setScratchValue(event) {
|
|
this.args.setScratchValue?.(event.target.value);
|
|
}
|
|
|
|
@action
|
|
setTwitterUrl(event) {
|
|
const {model, modelProperty} = this.args;
|
|
|
|
const newUrl = event.target.value;
|
|
|
|
// reset errors and validation
|
|
model.errors.remove(modelProperty);
|
|
model.hasValidated.removeObject(modelProperty);
|
|
|
|
if (!newUrl) {
|
|
// Clear out the Twitter url
|
|
model[modelProperty] = '';
|
|
this.args.setScratchValue?.(null);
|
|
return;
|
|
}
|
|
|
|
if (newUrl.match(/(?:twitter\.com\/)(\S+)/) || newUrl.match(/([a-z\d.]+)/i)) {
|
|
let username = [];
|
|
|
|
if (newUrl.match(/(?:twitter\.com\/)(\S+)/)) {
|
|
[, username] = newUrl.match(/(?:twitter\.com\/)(\S+)/);
|
|
} else {
|
|
[username] = newUrl.match(/([^/]+)\/?$/mi);
|
|
}
|
|
|
|
// check if username starts with http or www and show error if so
|
|
if (username.match(/^(http|www)|(\/)/) || !username.match(/^[a-z\d._]{1,15}$/mi)) {
|
|
const message = !username.match(/^[a-z\d._]{1,15}$/mi)
|
|
? 'Your Username is not a valid Twitter Username'
|
|
: 'The URL must be in a format like https://twitter.com/yourUsername';
|
|
|
|
model.errors.add(modelProperty, message);
|
|
model.hasValidated.pushObject(modelProperty);
|
|
return;
|
|
}
|
|
|
|
model[modelProperty] = `https://twitter.com/${username}`;
|
|
this.args.setScratchValue?.(null);
|
|
|
|
model.hasValidated.pushObject(modelProperty);
|
|
} else {
|
|
const message = 'The URL must be in a format like https://twitter.com/yourUsername';
|
|
model.errors.add(modelProperty, message);
|
|
model.hasValidated.pushObject(modelProperty);
|
|
return;
|
|
}
|
|
}
|
|
}
|